I like the equation of a sphere of radius R centered at the origin is given in Cartesian coordinates:
x*x + y*y + z*z = r*r
It is one of the first elements that helped me better understand mathematics and later the dynamics and theory of electromagnetic fields.
I did not find a graphical representation using python as accurately as possible without eliminating the discretion of the range from -1 and 1 and radius * radius = 1.
The main reason is the plot_surface from matplotlib python package.
This is output of my script:
[mythcat@desk ~]$ python sphere_xyz.py
[-1. -0.91666667 -0.83333333 -0.75 -0.66666667 -0.58333333
-0.5 -0.41666667 -0.33333333 -0.25 -0.16666667 -0.08333333
0. 0.08333333 0.16666667 0.25 0.33333333 0.41666667
0.5 0.58333333 0.66666667 0.75 0.83333333 0.91666667
1. ]
sphere_xyz.py:7: RuntimeWarning: invalid value encountered in sqrt
return np.sqrt(1-x**2 - y**2)
sphere_xyz.py:18: UserWarning: Z contains NaN values. This may result in rendering artifacts.
surface1 = ax.plot_surface(X2, Y2, -Z2,rstride=1, cstride=1, linewidth=0,antialiased=True)
sphere_xyz.py:19: UserWarning: Z contains NaN values. This may result in rendering artifacts.
surface2 = ax.plot_surface(X2, Y2, Z2,rstride=1, cstride=1, linewidth=0,antialiased=True)
The image result is this:
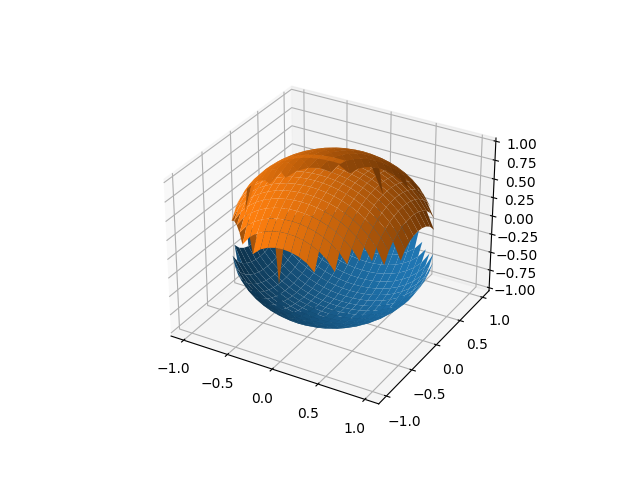
The source code is this:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.colors import LightSource
#@np.vectorize
def solve_Z2(x,y):
return np.sqrt(1-x**2 - y**2)
fig = plt.figure()
ax = fig.gca(projection='3d')
xrange2 = np.linspace(-1.0, 1.0, 25)
yrange2 = np.linspace(-1.0, 1.0, 25)
print(xrange2)
X2, Y2 = np.meshgrid(xrange2, yrange2)
Z2 = solve_Z2(X2, Y2)
surface1 = ax.plot_surface(X2, Y2, -Z2,rstride=1, cstride=1, linewidth=0,antialiased=True)
surface2 = ax.plot_surface(X2, Y2, Z2,rstride=1, cstride=1, linewidth=0,antialiased=True)
plt.show()