I used openai chat to test another issue with these python packages: ephem and matplotlib.
It seems that openai is limited to new changes in python packages, but it resolves quite well combinations of source code that it has corrected with defined errors. It can't really extract source code from general questions. Anyway, it is a very good help for a programmer in the initial phase of any project.
This source code show two diagrams about the solar system on a specific date:
import ephem
import matplotlib.pyplot as plt
# create an observer
obs = ephem.Observer()
# set the observer's location
obs.lat = '47.27' # latitude
obs.lon = '26.18' # longitude
obs.elevation = 307 # elevation (meters)
# set the date and time of the observation
obs.date = '2022/05/15 12:00:00' # date and time
# if you want you can use now() for real time data
# create the bodies
mercury = ephem.Mercury(obs)
venus = ephem.Venus(obs)
mars = ephem.Mars(obs)
jupiter = ephem.Jupiter(obs)
saturn = ephem.Saturn(obs)
uranus = ephem.Uranus(obs)
neptune = ephem.Neptune(obs)
pluto = ephem.Pluto(obs)
moon = ephem.Moon(obs)
# compute the position of each planet and the moon
mercury.compute(obs)
venus.compute(obs)
mars.compute(obs)
jupiter.compute(obs)
saturn.compute(obs)
uranus.compute(obs)
neptune.compute(obs)
pluto.compute(obs)
moon.compute(obs)
# extract ra and dec coordinates of each body
ra = [mercury.ra, venus.ra, mars.ra, jupiter.ra, saturn.ra, uranus.ra, neptune.ra, pluto.ra,moon.ra]
dec = [mercury.dec, venus.dec, mars.dec, jupiter.dec, saturn.dec, uranus.dec, neptune.dec, pluto.dec,moon.dec]
# convert ra,dec from radians to degrees
ra = [r*180/ephem.pi for r in ra]
dec = [d*180/ephem.pi for d in dec]
print(ra,dec)
# create a scatter plot of the positions
plt.scatter(ra, dec)
# add labels for each planet
plt.annotate('Mercury', (ra[0], dec[0]))
plt.annotate('Venus', (ra[1], dec[1]))
plt.annotate('Mars', (ra[2], dec[2]))
plt.annotate('Jupiter', (ra[3], dec[3]))
plt.annotate('Saturn', (ra[4], dec[4]))
plt.annotate('Uranus', (ra[5], dec[5]))
plt.annotate('Neptune', (ra[6], dec[6]))
plt.annotate('Pluto', (ra[7], dec[7]))
plt.annotate('Moon', (ra[8], dec[8]))
plt.xlabel("RA [degrees]")
plt.ylabel("Dec [degrees]")
# show the plot
plt.show()
# Set the figure size
plt.figure(figsize=(10, 10))
# Define the polar axis
ax = plt.subplot(111, projection='polar')
# Set the axis limits
ax.set_ylim(0, 36)
# Plot the Sun at the center
plt.scatter(0, 0, s=200, color='yellow')
mercury_distance = mercury.earth_distance
venus_distance= venus.earth_distance
mars_distance= mars.earth_distance
jupiter_distance= jupiter.earth_distance
saturn_distance= saturn.earth_distance
uranus_distance= uranus.earth_distance
neptune_distance= neptune.earth_distance
pluto_distance= pluto.earth_distance
moon_distance= moon.earth_distance
print(mercury_distance)
distance = [mercury_distance,venus_distance,mars_distance,jupiter_distance,saturn_distance,uranus_distance,neptune_distance,pluto_distance,moon_distance]
# Plot the planets
plt.scatter(ra[0], distance[0], s=20, color='green')
plt.scatter(ra[1], distance[1], s=50, color='orange')
plt.scatter(ra[2], distance[2], s=80, color='red')
plt.scatter(ra[3], distance[3], s=120, color='brown')
plt.scatter(ra[4], distance[4], s=150, color='tan')
plt.scatter(ra[5], distance[5], s=100, color='blue')
plt.scatter(ra[6], distance[6], s=80, color='cyan')
plt.scatter(ra[7], distance[7], s=40, color='purple')
plt.scatter(ra[8], distance[8], s=20, color='gray')
# add the labels for each planet
plt.annotate('Mercury',(ra[0], distance[0]),xytext=(ra[0], distance[0] - 2))
plt.annotate('Venus',(ra[1], distance[1]),xytext=(ra[1], distance[1] - 2))
plt.annotate('Mars',(ra[2], distance[2]),xytext=(ra[2], distance[2] - 2))
plt.annotate('Jupiter',(ra[3], distance[3]),xytext=(ra[3], distance[3] - 4))
plt.annotate('Saturn',(ra[4], distance[4]),xytext=(ra[4], distance[4] - 4))
plt.annotate('Uranus',(ra[5], distance[5]),xytext=(ra[5], distance[5] - 2))
plt.annotate('Neptune',(ra[6], distance[6]),xytext=(ra[6], distance[6] - 2))
plt.annotate('Pluto',(ra[7], distance[7]),xytext=(ra[7], distance[7] - 2))
plt.annotate('Moon',(ra[8], distance[8]),xytext=(ra[8], distance[8] - 2))
# Show the plot
plt.show()
This is the result of this source code:
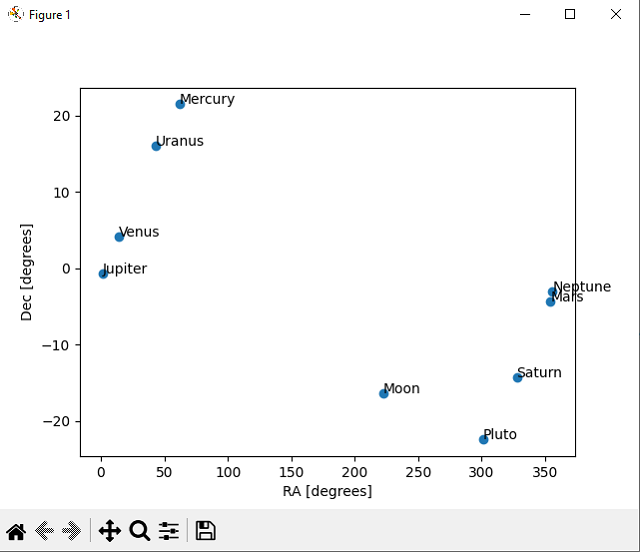
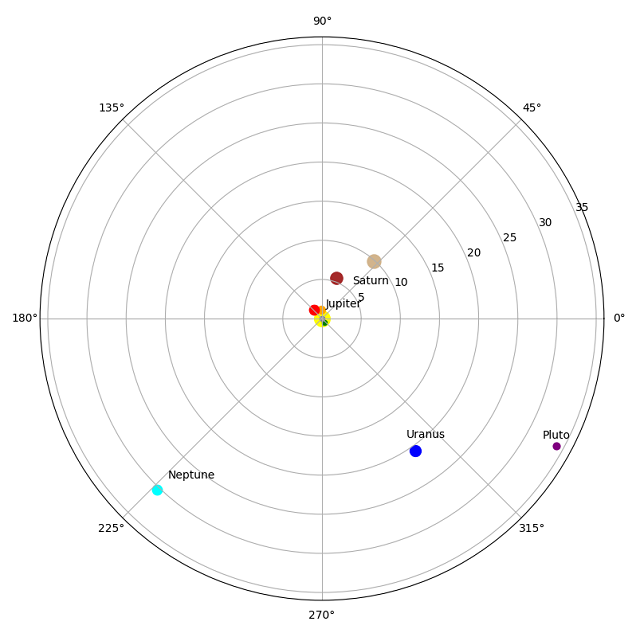