This script will add a submenu to the main Help menu with an icon with a folder that will open the explorer from the Windows operating system.
I have not solved the tooltip of this button, it is set to show a message about opening a URL.
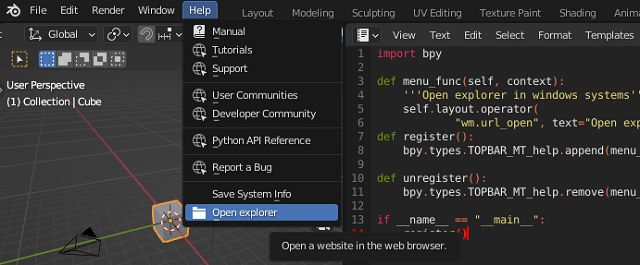
This is the source code:
import bpy
def menu_func(self, context):
'''Open explorer in windows systems'''
self.layout.operator(
"wm.url_open", text="Open explorer", icon='FILE_FOLDER').url = "C:/"
def register():
bpy.types.TOPBAR_MT_help.append(menu_func)
def unregister():
bpy.types.TOPBAR_MT_help.remove(menu_func)
if __name__ == "__main__":
register()
If you want to change the tooltip then need to create a class OpenOperator and wrapper for this operator function and set the bl_label and all you need to have it.
The menu_func will get the layout operator with all defined in the class OpenOperator and will set the tooltip with the text: Open explorer in windows systems.
See this new source code:
import bpy
def menu_func(self, context):
self.layout.operator(
OpenOperator.bl_idname, text="Open explorer", icon='FILE_FOLDER')
class OpenOperator(bpy.types.Operator):
"""Open explorer in windows systems"""
bl_idname = "wm.open_explorer"
bl_label = "Open explorer"
def execute(self, context):
bpy.ops.wm.url_open(url="C:/")
return {'FINISHED'}
def register():
bpy.utils.register_class(OpenOperator)
bpy.types.TOPBAR_MT_help.append(menu_func)
def unregister():
bpy.utils.unregister_class(OpenOperator)
bpy.types.TOPBAR_MT_help.remove(menu_func)
if __name__ == "__main__":
register()