So far I have added or branched used python scripts in the blender and created a UV texture for this use UV Smart projection.
The resulting script is quite large in content.
I thought I should create an addon to use and show you how to do it.
Let's follow the basic steps when working with python scripts in Blender 3D.
- go to the tag named: Scripting;
- use main menu to create a new script from Text - New;
- use main menu - Template - Python - Addon Add Object;
- save the script with a good name, I used: addon_catafest_add_branch.py;
- save the blend file with a good name: I used: addon_catafest_add_branch.blend;
See this screenshot:

After these changes you can modify in the template file the parts related to the names, descriptions and other elements that will be viewed in Blender 3D
Restart the Blender 3D software, open the python script with all changes and run it.
In the 3D Viewport area press the shortkeys: Shift + A and use Mesh menu to see this addon feature, see screenshot:
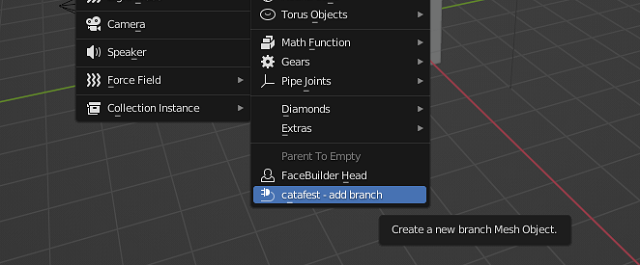
This is source code I used:
bl_info = {
"name": "New branch",
"author": "Your Name Here",
"version": (1, 0),
"blender": (3, 3, 0),
"location": "View3D > Add > Mesh > New Object",
"description": "Adds a new branch Mesh Object",
"warning": "",
"doc_url": "",
"category": "Add Mesh",
}
import bpy
from bpy.types import Operator
from bpy.props import FloatVectorProperty
from bpy_extras.object_utils import AddObjectHelper, object_data_add
from mathutils import Vector
def add_object(self, context):
scale_x = self.scale.x
scale_y = self.scale.y
verts = [
Vector((-1 * scale_x, 1 * scale_y, 0)),
Vector((1 * scale_x, 1 * scale_y, 0)),
Vector((1 * scale_x, -1 * scale_y, 0)),
Vector((-1 * scale_x, -1 * scale_y, 0)),
]
edges = []
faces = [[0, 1, 2, 3]]
mesh = bpy.data.meshes.new(name="New Object Mesh")
mesh.from_pydata(verts, edges, faces)
# useful for development when the mesh may be invalid.
# mesh.validate(verbose=True)
object_data_add(context, mesh, operator=self)
class OBJECT_OT_add_object(Operator, AddObjectHelper):
"""Create a new branch Mesh Object"""
bl_idname = "mesh.add_object"
bl_label = "Add Mesh Object"
bl_options = {'REGISTER', 'UNDO'}
scale: FloatVectorProperty(
name="scale",
default=(1.0, 1.0, 1.0),
subtype='TRANSLATION',
description="scaling",
)
def execute(self, context):
add_object(self, context)
return {'FINISHED'}
# Registration
def add_object_button(self, context):
self.layout.operator(
OBJECT_OT_add_object.bl_idname,
text="catafest - add branch",
icon='PLUGIN')
# This allows you to right click on a button and link to documentation
def add_object_manual_map():
url_manual_prefix = "https://docs.blender.org/manual/en/latest/"
url_manual_mapping = (
("bpy.ops.mesh.add_object", "scene_layout/object/types.html"),
)
return url_manual_prefix, url_manual_mapping
def register():
bpy.utils.register_class(OBJECT_OT_add_object)
bpy.utils.register_manual_map(add_object_manual_map)
bpy.types.VIEW3D_MT_mesh_add.append(add_object_button)
def unregister():
bpy.utils.unregister_class(OBJECT_OT_add_object)
bpy.utils.unregister_manual_map(add_object_manual_map)
bpy.types.VIEW3D_MT_mesh_add.remove(add_object_button)
if __name__ == "__main__":
register()