You need to install the PyQtWebEngine python package and to have the PyQt5 python package installed.
PyQt6 works in the same way as PyQt5 but with small differences ...
pip install PyQtWebEngine --user
Collecting PyQtWebEngine
Downloading PyQtWebEngine-5.15.6-cp37-abi3-win_amd64.whl (182 kB)
---------------------------------------- 182.7/182.7 kB 580.6 kB/s eta 0:00:00
...
---------------------------------------- 60.0/60.0 MB 655.7 kB/s eta 0:00:00
...
Installing collected packages: PyQtWebEngine-Qt5, PyQtWebEngine
Successfully installed PyQtWebEngine-5.15.6 PyQtWebEngine-Qt5-5.15.2
import sys
from PyQt5.QtWebEngineWidgets import QWebEngineView
from PyQt5.QtWidgets import QApplication, QWidget, QVBoxLayout
class MapWidget(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
# Create a QWebEngineView object to display the map
self.map = QWebEngineView()
self.map.setHtml("""
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
#map {
height: 100%;
}
</style>
</head>
<body>
<div id="map"></div>
<script src="https://openlayers.org/en/v4.6.5/build/ol.js"></script>
<script>
var map = new ol.Map({
target: 'map',
layers: [
new ol.layer.Tile({
source: new ol.source.OSM()
})
],
view: new ol.View({
center: ol.proj.fromLonLat([0, 0]),
zoom: 2
})
});
</script>
</body>
</html>
""")
# Create a QVBoxLayout to hold the map widget
layout = QVBoxLayout()
layout.addWidget(self.map)
self.setLayout(layout)
if __name__ == '__main__':
app = QApplication(sys.argv)
widget = MapWidget()
widget.show()
sys.exit(app.exec_())
The run of this python script will show a window with the OpenStreetMap map.
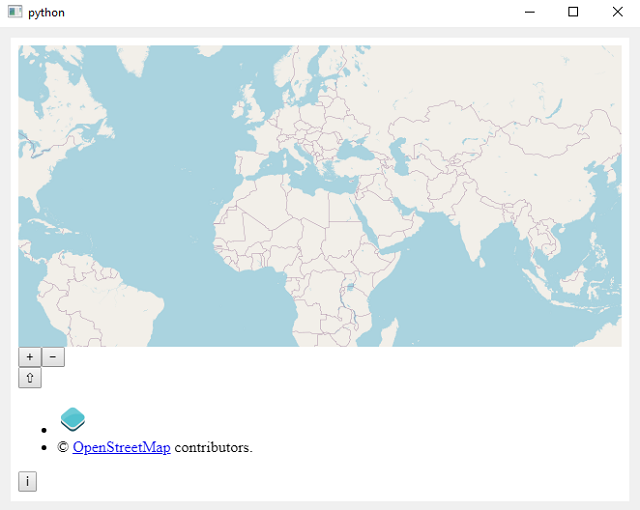