In this tutorial I will show you how to deal with the NASA A.P.I. and python programming language.
This source code was build and tested yesterday.
This is the source code:
import requests
from datetime import date
today_data = date.today()
today = today_data.strftime("%d%m%Y")
import urllib.parse
# set your API key from nasa https://api.nasa.gov/#NHATS
api_key = "... your A.P.I. key ..."
# this is a simple example to get one day image
base_url = "https://api.nasa.gov/planetary/apod"
# set the parameters for the API request
params = {
"api_key": api_key
}
# the request to the API
response = requests.get(base_url, params=params)
# get data
if response.status_code == 200:
# parse the response
data = response.json()
# print the image URL
print(data["url"])
# parse the URL
parsed_url = urllib.parse.urlparse(data["url"])
# extract the file name from the URL
file_name = parsed_url.path.split("/")[-1]
# save the image
response_image = requests.get(data["url"])
with open(today+'_'+file_name, "wb") as f:
f.write(response_image.content)
else:
# print the status code
print(response.status_code)
I run the source code and I get these two images ...
...
01/03/2023 01:06 AM 86,943 03012023_AllPlanets_Tezel_1080_annotated.jpg
01/03/2023 04:22 PM 553,426 03012023_KembleCascade_Lease_960.jpg
...
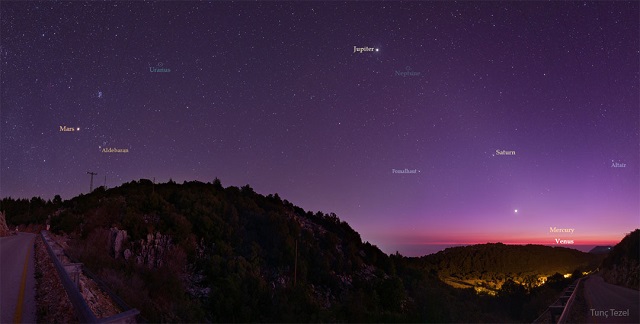
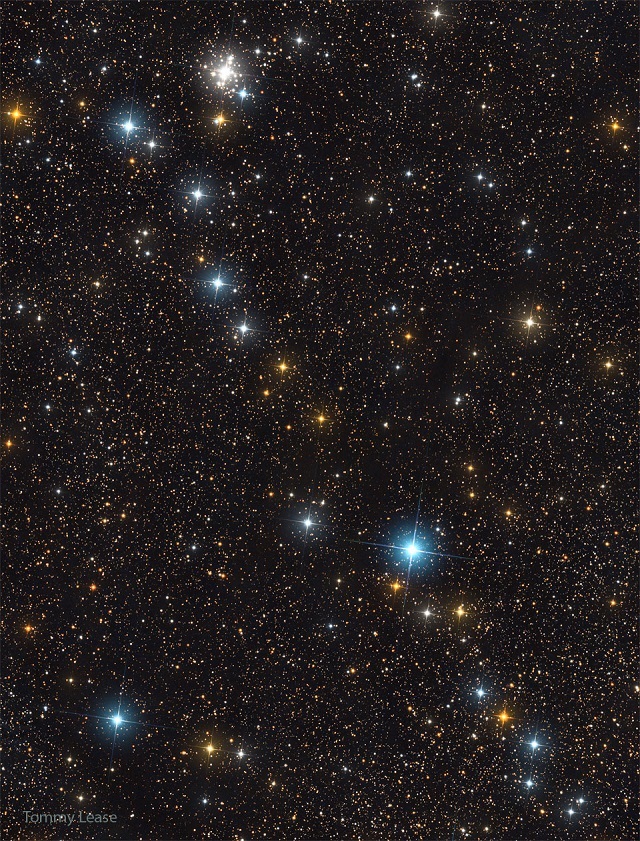