Let's make a test to see what is this:
>>> import PyQt5
>>> from PyQt5.QtOpenGL import *
>>> dir(PyQt5.QtOpenGL)
['QGL', 'QGLContext', 'QGLFormat', 'QGLWidget', '__doc__', '__file__', '__loader
__', '__name__', '__package__', '__spec__']
The QtOpenGL is used to take an OpenGL content and show into the PyQt5 application.You need to kknow how to use OpenGL API.
You can see the result of this example:
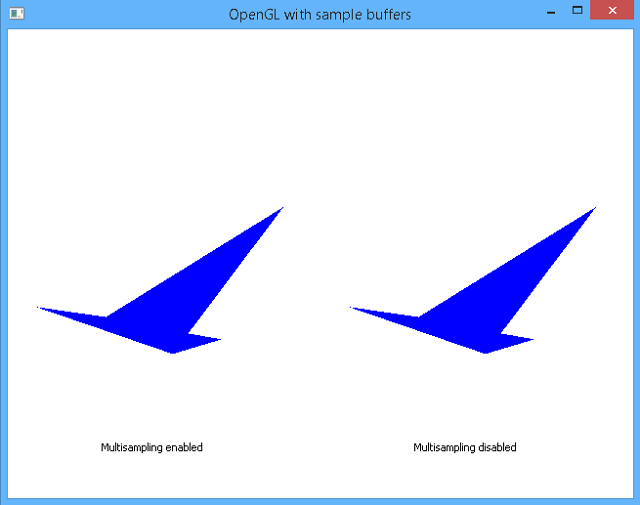
This is the source code for my example:
import sys
import math
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QColor
from PyQt5.QtWidgets import QApplication, QMessageBox
from PyQt5.QtOpenGL import QGL, QGLFormat, QGLWidget
try:
from OpenGL import GL
except ImportError:
app = QApplication(sys.argv)
QMessageBox.critical(None, "OpenGL samplebuffers",
"PyOpenGL must be installed to run this example.")
sys.exit(1)
# use to create OpenGL content
class GLWidget(QGLWidget):
GL_MULTISAMPLE = 0x809D
# rotation to 0
rot = 0.0
def __init__(self, parent):
super(GLWidget, self).__init__(QGLFormat(QGL.SampleBuffers), parent)
self.list_ = []
self.startTimer(40)
self.setWindowTitle("OpenGL with sample buffers")
def initializeGL(self):
GL.glMatrixMode(GL.GL_PROJECTION)
GL.glLoadIdentity()
GL.glOrtho( -.5, .5, .5, -.5, -1000, 1000)
GL.glMatrixMode(GL.GL_MODELVIEW)
GL.glLoadIdentity()
GL.glClearColor(1.0, 1.0, 1.0, 1.0)
self.makeObject()
def resizeGL(self, w, h):
GL.glViewport(0, 0, w, h)
def paintGL(self):
GL.glClear(GL.GL_COLOR_BUFFER_BIT | GL.GL_DEPTH_BUFFER_BIT)
GL.glMatrixMode(GL.GL_MODELVIEW)
GL.glPushMatrix()
GL.glEnable(GLWidget.GL_MULTISAMPLE)
GL.glTranslatef( -0.25, -0.10, 0.0)
GL.glScalef(0.75, 1.15, 0.0)
GL.glRotatef(GLWidget.rot, 0.0, 0.0, 1.0)
GL.glCallList(self.list_)
GL.glPopMatrix()
GL.glPushMatrix()
GL.glDisable(GLWidget.GL_MULTISAMPLE)
GL.glTranslatef(0.25, -0.10, 0.0)
GL.glScalef(0.75, 1.15, 0.0)
GL.glRotatef(GLWidget.rot, 0.0, 0.0, 1.0)
GL.glCallList(self.list_)
GL.glPopMatrix()
GLWidget.rot += 0.2
self.qglColor(Qt.black)
self.renderText(-0.35, 0.4, 0.0, "Multisampling enabled")
self.renderText(0.15, 0.4, 0.0, "Multisampling disabled")
def timerEvent(self, event):
self.update()
# create one object
def makeObject(self):
x1 = +0.05
y1 = +0.25
x2 = +0.15
y2 = +0.21
x3 = -0.25
y3 = +0.2
x4 = -0.1
y4 = +0.2
x5 = +0.25
y5 = -0.05
self.list_ = GL.glGenLists(1)
GL.glNewList(self.list_, GL.GL_COMPILE)
self.qglColor(Qt.blue)
self.geometry(GL.GL_POLYGON, x1, y1, x2, y2, x3, y3, x4, y4, x5, y5)
GL.glEndList()
# create geometry of object depend of render - GL_POLYGON
# used five points
def geometry(self, primitive, x1, y1, x2, y2, x3, y3, x4, y4, x5, y5):
GL.glBegin(primitive)
GL.glVertex2d(x1, y1)
GL.glVertex2d(x2, y2)
GL.glVertex2d(x3, y3)
GL.glVertex2d(x4, y4)
GL.glVertex2d(x5, y5)
GL.glEnd()
# start the application
if __name__ == '__main__':
app = QApplication(sys.argv)
my_format = QGLFormat.defaultFormat()
my_format.setSampleBuffers(True)
QGLFormat.setDefaultFormat(my_format)
if not QGLFormat.hasOpenGL():
QMessageBox.information(None, "OpenGL using samplebuffers",
"This system does not support OpenGL.")
sys.exit(0)
widget = GLWidget(None)
if not widget.format().sampleBuffers():
QMessageBox.information(None, "OpenGL using samplebuffers",
"This system does not have sample buffer support.")
sys.exit(0)
widget.resize(640, 480)
widget.show()
sys.exit(app.exec_())