In this tutorial, I will show you how to create a box from planes, see the screenshot:
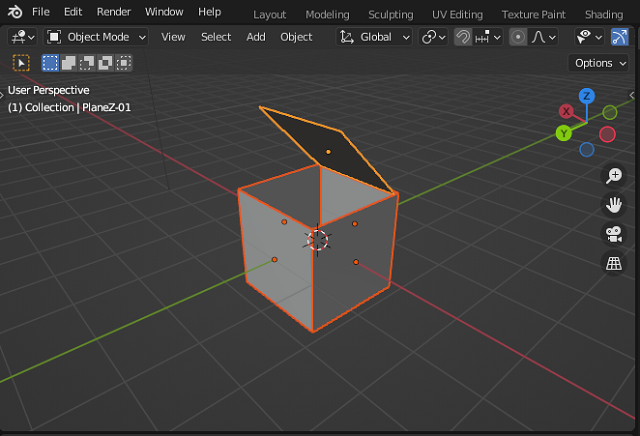
You can see I used the math python package and I created rotation by radians and rotation object for three custom planes.
This is the source code:
import bpy
import math
def DegToRad(angle):
"""convert to radians"""
return angle*(math.pi/180)
def RotOBJ(name, angles):
"""rotate obj to the specified angles"""
rotation = [DegToRad(angle) for angle in angles]
bpy.data.objects[name].rotation_euler = rotation
def create_plane_X(location, obj_name):
obj = bpy.ops.mesh.primitive_plane_add(size=2,
calc_uvs=True,
enter_editmode=False,
align='CURSOR',
location=location,
rotation=(0, 0, 0),
scale=(0,0,0)
)
# rename the object
bpy.context.object.name = obj_name
# return the object reference
return bpy.context.object
def create_plane_Y(location, obj_name):
obj = bpy.ops.mesh.primitive_plane_add(size=2,
calc_uvs=True,
enter_editmode=False,
align='CURSOR',
location=location,
rotation=(0, 0, 0),
scale=(0,0,0)
)
# rename the object
bpy.context.object.name = obj_name
# return the object reference
return bpy.context.object
def create_plane_Z(location, obj_name):
obj = bpy.ops.mesh.primitive_plane_add(size=2,
calc_uvs=True,
enter_editmode=False,
align='CURSOR',
location=location,
rotation=(0, 0, 0),
scale=(0,0,0)
)
# rename the object
bpy.context.object.name = obj_name
# return the object reference
return bpy.context.object
n = 1
planeX001 = create_plane_X((0,-1,0), "PlaneX-{:02d}".format(n))
RotOBJ(planeX001.name, [-90, 0, 0])
planeX002 = create_plane_X((0,1,0), "PlaneX-{:02d}".format(n))
RotOBJ(planeX002.name, [-90, -0, 0])
planeY001 = create_plane_Y((-1,0,0), "PlaneY-{:02d}".format(n))
RotOBJ(planeY001.name, [0, -90, 0])
planeY002 = create_plane_Y((1,0,0), "PlaneY-{:02d}".format(n))
RotOBJ(planeY002.name, [0, -90, 0])
planeZ001 = create_plane_Z((0,-0.25,1.66), "PlaneZ-{:02d}".format(n))
RotOBJ(planeZ001.name, [45, 0, 0])