In the last tutorial, we exemplified with the default template from Blender 3D how to create a panel in the Object area.
Today I will show you how to modify this panel with some useful elements for developing an addon.
The purpose of the old tutorial on this is the differences and changes that must be made to the template source code to introduce the following functions:
StringProperty(
BoolProperty(
IntProperty(
IntVectorProperty(
FloatProperty(
FloatVectorProperty(
BoolVectorProperty(
Some arguments need to be modified to have different input data, see the selection of colors in the attached image:
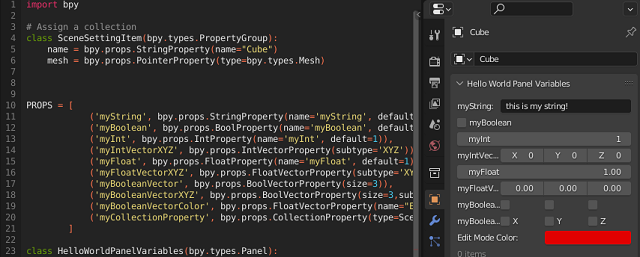
I commented on the source code areas in the template and added my changes:
The class also called SceneSettingItem and CollectionProperty is currently being tested and is not finalized to be implemented, it can be seen in panel: 0 items.
It can be seen that any defined class must be registered and unregistered
Here is the source code used to get the new screenshot changes:
import bpy
# Assign a collection
class SceneSettingItem(bpy.types.PropertyGroup):
name = bpy.props.StringProperty(name="Cube")
mesh = bpy.props.PointerProperty(type=bpy.types.Mesh)
PROPS = [
('myString', bpy.props.StringProperty(name='myString', default='this is my string!')),
('myBoolean', bpy.props.BoolProperty(name='myBoolean', default=False)),
('myInt', bpy.props.IntProperty(name='myInt', default=1)),
('myIntVectorXYZ', bpy.props.IntVectorProperty(subtype='XYZ')),
('myFloat', bpy.props.FloatProperty(name='myFloat', default=1)),
('myFloatVectorXYZ', bpy.props.FloatVectorProperty(subtype='XYZ')),
('myBooleanVector', bpy.props.BoolVectorProperty(size=3)),
('myBooleanVectorXYZ', bpy.props.BoolVectorProperty(size=3,subtype='XYZ')),
('myBooleanVectorColor', bpy.props.FloatVectorProperty(name="Edit Mode Color", subtype='COLOR', default=(0.76, 0.0, 0.0), size=3, min=0, max=1)),
('myCollectionProperty', bpy.props.CollectionProperty(type=SceneSettingItem)),
]
class HelloWorldPanelVariables(bpy.types.Panel):
"""Creates a Panel in the Object properties window"""
bl_label = "Hello World Panel Variables"
bl_idname = "OBJECT_PT_hello"
bl_space_type = 'PROPERTIES'
bl_region_type = 'WINDOW'
bl_context = "object"
# def draw(self, context):
# layout = self.layout
# obj = context.object
# row = layout.row()
# row.label(text="Hello world!", icon='WORLD_DATA')
# row = layout.row()
# row.label(text="Active object is: " + obj.name)
# row = layout.row()
# row.prop(obj, "name")
# row = layout.row()
# row.operator("mesh.primitive_cube_add")
def draw(self, context):
col = self.layout.column()
for (prop_name, _) in PROPS:
row = col.row()
row.prop(context.scene, prop_name)
def register():
bpy.utils.register_class(SceneSettingItem)
bpy.utils.register_class(HelloWorldPanelVariables)
for (prop_name, prop_value) in PROPS:
setattr(bpy.types.Scene, prop_name, prop_value)
def unregister():
bpy.utils.unregister_class(SceneSettingItem)
bpy.utils.unregister_class(HelloWorldPanelVariables)
for (prop_name, _) in PROPS:
delattr(bpy.types.Scene, prop_name)
if __name__ == "__main__":
register()