I used the google-api-python-client from here with OAuth service.
You need to create a project and add the blogger API to use it.
For credentials, I used OAuth with the JSON file from google.
This is the source code:
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from __future__ import print_function
__author__ = 'Catalin George Festila ( catafest , mythcat )'
import sys
from oauth2client import client
from googleapiclient import sample_tools
def main(argv):
# Authenticate and construct service.
service, flags = sample_tools.init(
argv, 'blogger', 'v3', __doc__, __file__,
scope='https://www.googleapis.com/auth/blogger')
try:
users = service.users()
# Retrieve this user's profile information
thisuser = users.get(userId='self').execute()
print('This user\'s display name is: %s' % thisuser['displayName'])
blogs = service.blogs()
# Retrieve the list of Blogs this user has write privileges on
thisusersblogs = blogs.listByUser(userId='self').execute()
for blog in thisusersblogs['items']:
print('The blog named \'%s\' is at: %s' % (blog['name'], blog['url']))
posts = service.posts()
# List the posts for each blog this user has
for blog in thisusersblogs['items']:
print('The posts for %s:' % blog['name'])
request = posts.list(blogId=blog['id'])
while request != None:
posts_doc = request.execute()
if 'items' in posts_doc and not (posts_doc['items'] is None):
for post in posts_doc['items']:
print(' %s (%s)' % (post['title'], post['url']))
request = posts.list_next(request, posts_doc)
except client.AccessTokenRefreshError:
print ('The credentials have been revoked or expired, please re-run'
'the application to re-authorize')
if __name__ == '__main__':
main(sys.argv)
The content of the folder project named google_api_python.c:\Python364\google_api_python>dir
06/24/2018 08:27 PM 1,096 blogger.dat
06/24/2018 07:49 PM 2,599 blogger.py
06/24/2018 08:24 PM 309 client_secrets.json
06/24/2018 08:26 PM 76 debug.log
4 File(s) 4,080 bytes
2 Dir(s) 201,382,006,784 bytes free
You need to add the client_id and client_secret from google project into file client_secrets.json.Run the python script:
c:\Python364\google_api_python>python.exe blogger.py
The google will ask you about the authentification and will run the script: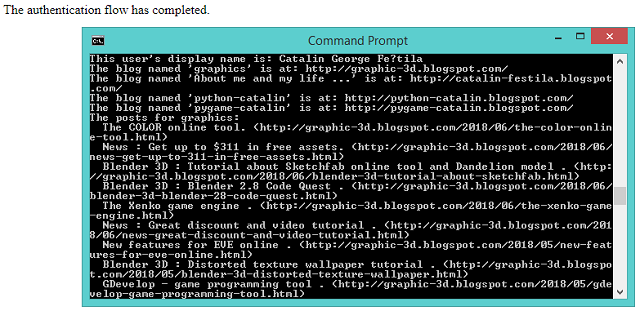
Google show us all the infos about this project into dashboard:
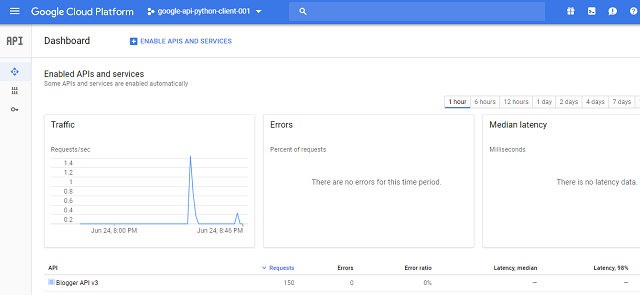