The source code uses the QTabWidget and create two Tab: FirstTab and TabTwo.
I add for each tab two labels: tab_one_label_one, tab_one_label_two,tab_two_label_one and tab_two_label_two.
For layouts, I used: vboxLayout with first_layout and second_layout.
The result of my running code is this:
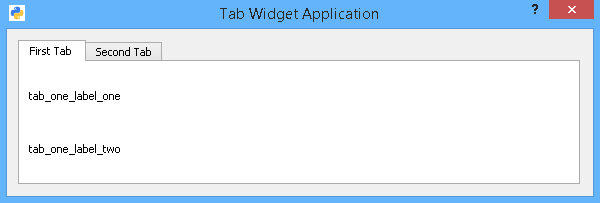
The rest of the source code is simple:
import sys
from PyQt5.QtGui import QIcon
from PyQt5.QtWidgets import QDialog, QApplication, QWidget
from PyQt5.QtWidgets import QVBoxLayout, QTabWidget, QLabel
class TabDialog(QDialog):
def __init__(self):
super().__init__()
self.setWindowTitle("Tab Widget Application")
self.setWindowIcon(QIcon("icon.png"))
tabwidget = QTabWidget()
tabwidget.addTab(FirstTab(), "First Tab")
tabwidget.addTab(TabTwo(), "Second Tab")
vboxLayout = QVBoxLayout()
vboxLayout.addWidget(tabwidget)
self.setLayout(vboxLayout)
class FirstTab(QWidget):
def __init__(self):
super().__init__()
tab_one_label_one = QLabel("tab_one_label_one")
tab_one_label_two = QLabel("tab_one_label_two")
first_layout = QVBoxLayout()
first_layout.addWidget(tab_one_label_one)
first_layout.addWidget(tab_one_label_two)
self.setLayout(first_layout)
class TabTwo(QWidget):
def __init__(self):
super().__init__()
tab_two_label_one = QLabel("tab_two_label_one")
tab_two_label_two = QLabel("tab_two_label_two")
second_layout = QVBoxLayout()
second_layout.addWidget(tab_two_label_one)
second_layout.addWidget(tab_two_label_two)
self.setLayout(second_layout)
if __name__ == "__main__":
app = QApplication(sys.argv)
tabdialog = TabDialog()
tabdialog.show()
app.exec()