First i wanted to make a simple example. Then I saw George Oliver googlegrups question. This made me change my little script.Official Site address is:
www.pyglet.org.
Documentation of this module is to:
www.pyglet.org/documentation.html.
There is also a Google group for this mode where you can ask questions.
My example does nothing more than:
- Create a fereasta;
- To put a background color;
- Displaying an image.
Image i use it, name it "20000.jpg" is this:
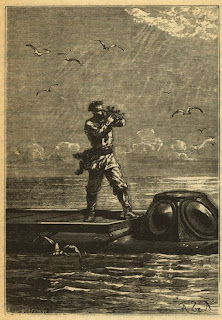
Put in folder with your script.
In the future I will come with more complex examples.
Change the following sequence of lines of code example:
win.clear ()
img1.blit (5, 5)
You will see that the order matters for pyglet.
Who studied OpenGL knows why.
import pyglet
from pyglet.gl import *
#set colors with red,green,blue,alpha values
colors = {
'playfield' : (255, 255, 255, 255),
'red' : (255, 0, 0, 255,0),
'sub_red' : (255, 50, 20, 255),
'green' : (0, 255, 0, 255),
'sub_green' : (20, 255, 50, 255),
'blue' : (0, 0, 255, 255),
'sub_blue' : (20, 50, 255, 255)}
#give img1 the place of image
img1= pyglet.image.load('20000.jpg')
#w , h the size of image
w = img1.width+15
h = img1.height+10
# create the 640x480 window size
win = pyglet.window.Window(640, 480, resizable=True, visible=True)
#or use next line to see with size of image
#win=pyglet.window.Window(width=w, height=h)
# set caption of window
win.set_caption("TEST")
#use * to unpack tuple and set background color
pyglet.gl.glClearColor(*colors['red'])
@win.event
def on_draw():
win.clear()
img1.blit(5, 5)
# to run application
pyglet.app.run()
If you use the code line:
win = pyglet.window.Window(640, 480, resizable=True, visible=True)
you see:
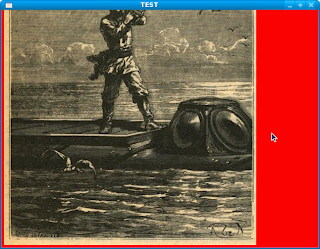
If you use the code line:
win=pyglet.window.Window(width=w, height=h)
you see: