Here is a Python script for Krita that will help you read the Python functions of a document.
Open a new document, create a python script, and add the source code. Use the main menu Tools - Scripts - Ten Scripts to choose the place where the script exists and the combination of keys for execution.
This is the source code:
from PyQt5.QtWidgets import QWidget, QVBoxLayout, QTreeWidget, QTreeWidgetItem, QTextEdit
from krita import *
class LayerContentDialog(QWidget):
def __init__(self, content):
super().__init__()
self.setWindowTitle("Conținutul preluat cu dir():")
self.setGeometry(100, 100, 800, 600)
main_layout = QVBoxLayout()
tree_widget = QTreeWidget()
tree_widget.setHeaderLabels(["Nume", "Tip"])
self.add_items_recursive(tree_widget, content)
main_layout.addWidget(tree_widget)
close_button = QPushButton("Închide")
close_button.clicked.connect(self.close)
main_layout.addWidget(close_button)
self.setLayout(main_layout)
def add_items_recursive(self, tree_widget, items, parent_item=None):
for item in items:
if isinstance(item, str):
if parent_item:
item_widget = QTreeWidgetItem(parent_item, [item, type(getattr(doc, item)).__name__])
help_text = "\n".join(dir(getattr(doc, item)))
item_widget.addChild(QTreeWidgetItem([help_text]))
else:
item_widget = QTreeWidgetItem(tree_widget, [item, type(getattr(doc, item)).__name__])
help_text = "\n".join(dir(getattr(doc, item)))
item_widget.addChild(QTreeWidgetItem([help_text]))
elif isinstance(item, type):
parent = QTreeWidgetItem(tree_widget, [item.__name__, "Modul"])
self.add_items_recursive(tree_widget, dir(item), parent)
doc = Krita.instance().activeDocument()
layerContents = dir(doc)
dialog = LayerContentDialog(layerContents)
dialog.show()
The result for this script is this:
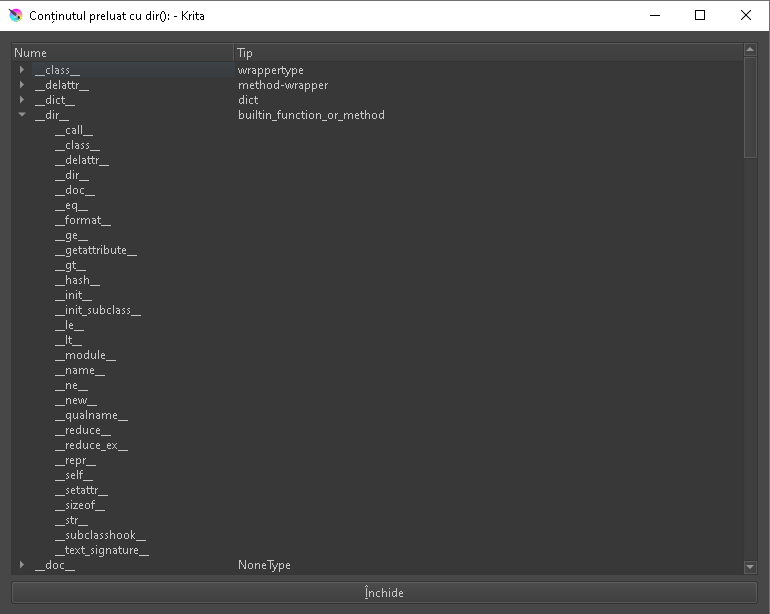
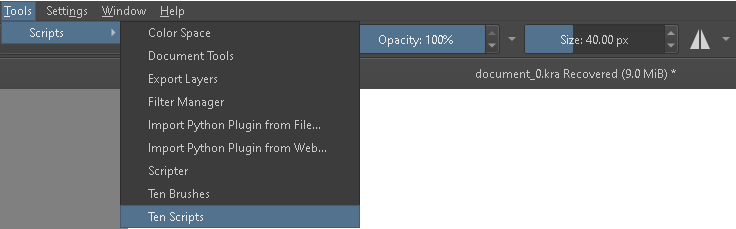
You can use the main menu Tools - Scripts - Scripter to write, test, save, and run the source code.
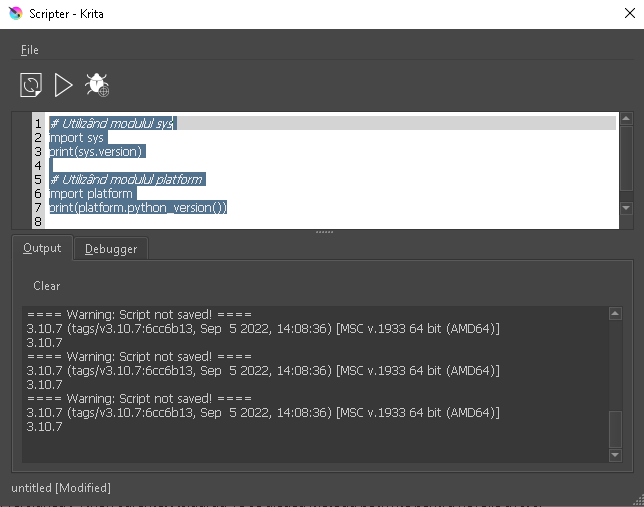