I try to create a simple example in order to have a good look at how can be used.
The main goal was to understand how can have the basic elements of QPainter.
The result of my example is this:
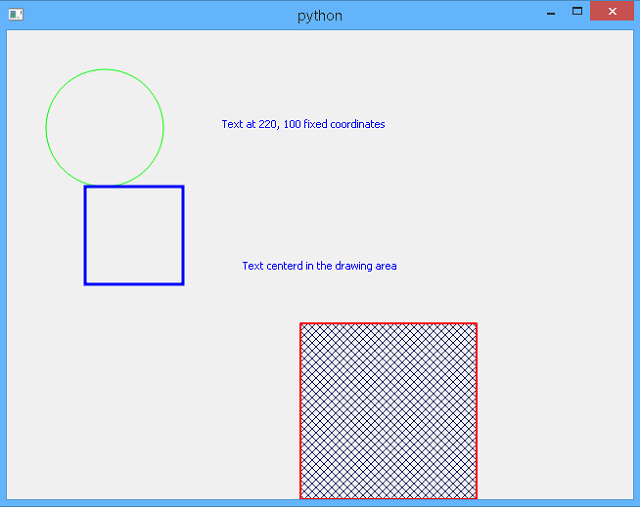
Here is my example with all commented lines for a good approach:
import sys
from PyQt5 import QtGui, QtWidgets
from PyQt5.QtGui import QPainter, QBrush, QColor
from PyQt5.QtCore import Qt, QPoint
class My_QPainter(QtWidgets.QWidget):
def paintEvent(self, event):
# create custom QPainter
my_painter = QtGui.QPainter()
# start and set my_painter
my_painter.begin(self)
my_painter.setRenderHint(my_painter.TextAntialiasing, True)
my_painter.setRenderHint(my_painter.Antialiasing, True)
#set color for pen by RGB
my_painter.setPen(QtGui.QColor(0,0,255))
# draw a text on fixed coordinates
my_painter.drawText(220,100, "Text at 220, 100 fixed coordinates")
# draw a text in the centre of my_painter
my_painter.drawText(event.rect(), Qt.AlignCenter, "Text centerd in the drawing area")
#set color for pen by Qt color
my_painter.setPen(QtGui.QPen(Qt.green, 1))
# draw a ellipse
my_painter.drawEllipse(QPoint(100,100),60,60)
# set color for pen by property
my_painter.setPen(QtGui.QPen(Qt.blue, 3, join = Qt.MiterJoin))
# draw a rectangle
my_painter.drawRect(80,160,100,100)
# set color for pen by Qt color
my_painter.setPen(QtGui.QPen(Qt.red, 2))
# set brush
my_brush = QBrush(QColor(33, 33, 100, 255), Qt.DiagCrossPattern)
my_painter.setBrush(my_brush)
# draw a rectangle and fill with the brush
my_painter.drawRect(300, 300,180, 180)
my_painter.end()
# create application
app = QtWidgets.QApplication(sys.argv)
# create the window application from class
window = My_QPainter()
# show the window
window.show()
# default exit
sys.exit(app.exec_())