This source code is a try to solve the video cutting background.
import cv2
from cv2 import *
import numpy as np
cap = cv2.VideoCapture("avi_test_001.avi")
while(True):
ret, img = cap.read()
mask = np.zeros(img.shape[:2],np.uint8)
bgdModel = np.zeros((1,65),np.float64)
fgdModel = np.zeros((1,65),np.float64)
rect = (50,50,450,290)
cv2.grabCut(img,mask,rect,bgdModel,fgdModel,5,cv2.GC_INIT_WITH_RECT)
mask2 = np.where((mask==2)|(mask==0),0,1).astype('uint8')
img = img*mask2[:,:,np.newaxis]
cv2.imshow('frame',img)
if 0xFF & cv2.waitKey(5) == 27:
break
cap.release()
cv2.destroyAllWindows()
Is a blog about python programming language. You can see my work with python programming language, tutorials and news.
Wednesday, July 6, 2016
Saturday, June 25, 2016
OpenGL and OpenCV with python 2.7 - part 002.
I deal today with opencv and I fix some of my errors.
One is this error I got with cv2.VideoCapture. When I try to used with load video and createBackgroundSubtractorMOG2() i got this:
cv2.error: C:\builds\master_PackSlaveAddon-win64-vc12-static\opencv\modules\highgui\src\window.cpp:281: error: (-215) size.width<0 amp="" cv::imshow="" function="" i="" in="" size.height="">0>
You need also to have opencv_ffmpeg310.dll and opencv_ffmpeg310_64.dll into your Windows C:\Windows\System32, this will help me to play videos.
Now make sure you have the opencv version 3.1.0 because opencv come with some changes over python.
C:\Python27\python
Python 2.7.8 (default, Jun 30 2014, 16:08:48) [MSC v.1500 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>import cv2
>>>print cv2.__version__
3.1.0
You can take some infos from about opencv python module - cv2 with:
>>>cv2.getBuildInformation()
...
>>>cv2.getCPUTickCount()
...
>>>print cv2.getNumberOfCPUs()
...
>>>print cv2.ocl.haveOpenCL()
True
You can also see some error by disable OpenCL:
>>>cv2.ocl.setUseOpenCL(False)
>>>print cv2.ocl.useOpenCL()
False
Now will show you how to use webcam gray and color , and play one video:
webcam color
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
while(True):
ret, frame = cap.read()
cv2.imshow('frame',frame)
if 0xFF & cv2.waitKey(5) == 27:
break
cap.release()
cv2.destroyAllWindows()
webcam gray
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
while(True):
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
cv2.imshow('frame',gray)
if 0xFF & cv2.waitKey(5) == 27:
break
cap.release()
cv2.destroyAllWindows()
play video
import cv2
from cv2 import *
capture = cv2.VideoCapture("avi_test_001.avi")
while True:
ret, img = capture.read()
cv2.imshow('some', img)
if 0xFF & cv2.waitKey(5) == 27:
break
cv2.destroyAllWindows()
One is this error I got with cv2.VideoCapture. When I try to used with load video and createBackgroundSubtractorMOG2() i got this:
cv2.error: C:\builds\master_PackSlaveAddon-win64-vc12-static\opencv\modules\highgui\src\window.cpp:281: error: (-215) size.width<0 amp="" cv::imshow="" function="" i="" in="" size.height="">0>
You need also to have opencv_ffmpeg310.dll and opencv_ffmpeg310_64.dll into your Windows C:\Windows\System32, this will help me to play videos.
Now make sure you have the opencv version 3.1.0 because opencv come with some changes over python.
C:\Python27\python
Python 2.7.8 (default, Jun 30 2014, 16:08:48) [MSC v.1500 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>import cv2
>>>print cv2.__version__
3.1.0
You can take some infos from about opencv python module - cv2 with:
>>>cv2.getBuildInformation()
...
>>>cv2.getCPUTickCount()
...
>>>print cv2.getNumberOfCPUs()
...
>>>print cv2.ocl.haveOpenCL()
True
You can also see some error by disable OpenCL:
>>>cv2.ocl.setUseOpenCL(False)
>>>print cv2.ocl.useOpenCL()
False
Now will show you how to use webcam gray and color , and play one video:
webcam color
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
while(True):
ret, frame = cap.read()
cv2.imshow('frame',frame)
if 0xFF & cv2.waitKey(5) == 27:
break
cap.release()
cv2.destroyAllWindows()
webcam gray
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
while(True):
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
cv2.imshow('frame',gray)
if 0xFF & cv2.waitKey(5) == 27:
break
cap.release()
cv2.destroyAllWindows()
play video
import cv2
from cv2 import *
capture = cv2.VideoCapture("avi_test_001.avi")
while True:
ret, img = capture.read()
cv2.imshow('some', img)
if 0xFF & cv2.waitKey(5) == 27:
break
cv2.destroyAllWindows()
Posted by
Cătălin George Feștilă
Labels:
2.7,
2016,
cv2,
matplotlib,
numpy,
OpenCV,
opengl,
pyopengl,
python
Wednesday, June 22, 2016
OpenGL and OpenCV with python 2.7 - part 001.
First you need to know what version of python you use.
You need also to download the OpenCV version 3.0 from here.
Then run the executable into your folder and get cv2.pyd file from \opencv\build\python\2.7\x64 and paste to \Python27\Lib\site-packages.
If you use then use 32 bit python version then use this path: \opencv\build\python\2.7\x86.
Use pip to install next python modules:
Let's see how is working OpenGL:
You can also use dir(module) to see more. You can import all from GL, GLU and GLUT.
If you are very good with python OpenGL module then you can import just like this example:
Most of this OpenGL need to have a valid OpenGL rendering context.
For example you can test it with WGL ( WGL or Wiggle is an API between OpenGL and the windowing system interface of Microsoft Windows):
Now , let's see the OpenCV python module with s=one simple webcam python script:

C:\Python27>python
Python 2.7.8 (default, Jun 30 2014, 16:08:48) [MSC v.1500 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>
You need also to download the OpenCV version 3.0 from here.
Then run the executable into your folder and get cv2.pyd file from \opencv\build\python\2.7\x64 and paste to \Python27\Lib\site-packages.
If you use then use 32 bit python version then use this path: \opencv\build\python\2.7\x86.
Use pip to install next python modules:
C:\Python27\Scripts>pip install PyOpenGL
...
C:\Python27\Scripts>pip install numpy
...
C:\Python27\Scripts>pip install matplotlib
...
Let's see how is working OpenGL:
C:\Python27>python
Python 2.7.8 (default, Jun 30 2014, 16:08:48) [MSC v.1500 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import OpenGL
>>> import numpy
>>> import matplotlib
>>> import cv2
>>> from OpenGL import *
>>> from numpy import *
>>> from matplotlib import *
>>> from cv2 import *
You can also use dir(module) to see more. You can import all from GL, GLU and GLUT.
>>> dir(OpenGL)
['ALLOW_NUMPY_SCALARS', 'ARRAY_SIZE_CHECKING', 'CONTEXT_CHECKING', 'ERROR_CHECKING', 'ERROR_LOGGING', 'ERROR_ON_COPY', 'FORWARD_COMPATIBLE_ONLY', 'FULL_LOGGING', 'FormatHandler', 'MODULE_ANNOTATIONS', 'PlatformPlugin', 'SIZE_1_ARRAY_UNPACK', 'STORE_POINTERS', 'UNSIGNED_BYTE_IMAGES_AS_STRING', 'USE_ACCELERATE', 'WARN_ON_FORMAT_UNAVAILABLE', '__builtins__', '__doc__', '__file__', '__name__', '__package__', '__path__', '__version__', '_bi', 'environ_key', 'os', 'plugins', 'sys', 'version']
>>> from OpenGL.GL import *
>>> from OpenGL.GLU import *
>>> from OpenGL.GLUT import *
>>> from OpenGL.WGL import *
If you are very good with python OpenGL module then you can import just like this example:
>>> from OpenGL.arrays import ArrayDatatype
>>> from OpenGL.GL import (GL_ARRAY_BUFFER, GL_COLOR_BUFFER_BIT,
... GL_COMPILE_STATUS, GL_FALSE, GL_FLOAT, GL_FRAGMENT_SHADER,
... GL_LINK_STATUS, GL_RENDERER, GL_SHADING_LANGUAGE_VERSION,
... GL_STATIC_DRAW, GL_TRIANGLES, GL_TRUE, GL_VENDOR, GL_VERSION,
... GL_VERTEX_SHADER, glAttachShader, glBindBuffer, glBindVertexArray,
... glBufferData, glClear, glClearColor, glCompileShader,
... glCreateProgram, glCreateShader, glDeleteProgram,
... glDeleteShader, glDrawArrays, glEnableVertexAttribArray,
... glGenBuffers, glGenVertexArrays, glGetAttribLocation,
... glGetProgramInfoLog, glGetProgramiv, glGetShaderInfoLog,
... glGetShaderiv, glGetString, glGetUniformLocation, glLinkProgram,
... glShaderSource, glUseProgram, glVertexAttribPointer)
Most of this OpenGL need to have a valid OpenGL rendering context.
For example you can test it with WGL ( WGL or Wiggle is an API between OpenGL and the windowing system interface of Microsoft Windows):
>>> import OpenGL
>>> from OpenGL import *
>>> from OpenGL import WGL
>>> print WGL.wglGetCurrentDC()
None
Now , let's see the OpenCV python module with s=one simple webcam python script:
import numpy as np
import cv2
cap = cv2.VideoCapture(0)
while(True):
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
cv2.imshow('frame',gray)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
This is result of my webcam:
Posted by
Cătălin George Feștilă
Labels:
2.7,
2016,
cv2,
matplotlib,
numpy,
OpenCV,
opengl,
pyopengl,
python
Tuesday, June 21, 2016
Scrapy python module - part 001.
To install pip under python 2.7.8, securely download get-pip.py into Python27 folder.
Use this command:
Some of python modules are installed:
Now you need to install win32api with this python module:
... and test scrapy bench:
Into the next tutorial I will try to use scrapy.
If you have some ideas about how to do the next step just send me one comment.
Use this command:
C:\Python27\python.exe get-pip.py
...
C:\Python27\Scripts>pip2.7.exe install urllib3
C:\Python27\Scripts>pip2.7 install requests
C:\Python27\Scripts>pip install Scrapy
Some of python modules are installed:
Successfully built PyDispatcher pycparser
Installing collected packages: cssselect, queuelib, six, enum34, ipaddress, idna, pycparser, cffi, pyasn1, cryptography, pyOpenSSL, w3lib, lxml, parsel, PyDispatcher, zope.interface, Twisted, attrs, pyasn1-modules, service-identity, Scrapy
Successfully installed PyDispatcher-2.0.5 Scrapy-1.1.0 Twisted-16.2.0 attrs-16.0.0 cffi-1.7.0 cryptography-1.4 cssselect-0.9.2 enum34-1.1.6 idna-2.1 ipaddress-1.0.16 lxml-3.6.0 parsel-1.0.2 pyOpenSSL-16.0.0 pyasn1-0.1.9 pyasn1-modules-0.0.8 pycparser-2.14 queuelib-1.4.2 service-identity-16.0.0 six-1.10.0 w3lib-1.14.2 zope.interface-4.2.0
>>> print scrapy.version_info
(1, 1, 0)
>>> help(scrapy)
PACKAGE CONTENTS
_monkeypatches
cmdline
command
commands (package)
conf
contracts (package)
contrib (package)
contrib_exp (package)
core (package)
crawler
downloadermiddlewares (package)
dupefilter
dupefilters
exceptions
exporters
extension
extensions (package)
http (package)
interfaces
item
link
linkextractor
linkextractors (package)
loader (package)
log
logformatter
mail
middleware
pipelines (package)
project
resolver
responsetypes
selector (package)
settings (package)
shell
signalmanager
signals
spider
spiderloader
spidermanager
spidermiddlewares (package)
spiders (package)
squeue
squeues
stats
statscol
statscollectors
telnet
utils (package)
xlib (package)
...
C:\Python27\c:\Python27\Scripts\scrapy.exe startproject test_scrapy
New Scrapy project 'test_scrapy', using template directory 'c:\\python27\\lib\\site-packages\\scrapy\\templates\\project', created in:
C:\Python27\test_scrapy
You can start your first spider with:
cd test_scrapy
scrapy genspider example example.com
C:\Python27\cd test_scrapy
C:\Python27\test_scrapy>tree
Folder PATH listing
Volume serial number is 9A67-3A80
C:.
└───test_scrapy
└───spiders
Now you need to install win32api with this python module:
pip install pypiwin32
...
Downloading pypiwin32-219-cp27-none-win_amd64.whl (7.3MB)
100% |################################| 7.3MB 61kB/s
Installing collected packages: pypiwin32
Successfully installed pypiwin32-219
... and test scrapy bench:
C:\Python27\Scripts\scrapy.exe bench
2016-06-21 22:45:20 [scrapy] INFO: Scrapy 1.1.0 started (bot: scrapybot)
2016-06-21 22:45:20 [scrapy] INFO: Overridden settings: {'CLOSESPIDER_TIMEOUT': 10, 'LOG_LEVEL': 'INFO', 'LOGSTATS_INTERVAL': 1}
2016-06-21 22:45:39 [scrapy] INFO: Enabled extensions:
['scrapy.extensions.closespider.CloseSpider',
'scrapy.extensions.logstats.LogStats',
'scrapy.extensions.telnet.TelnetConsole',
'scrapy.extensions.corestats.CoreStats']
2016-06-21 22:45:46 [scrapy] INFO: Enabled downloader middlewares:
['scrapy.downloadermiddlewares.httpauth.HttpAuthMiddleware',
'scrapy.downloadermiddlewares.downloadtimeout.DownloadTimeoutMiddleware',
'scrapy.downloadermiddlewares.useragent.UserAgentMiddleware',
'scrapy.downloadermiddlewares.retry.RetryMiddleware',
'scrapy.downloadermiddlewares.defaultheaders.DefaultHeadersMiddleware',
'scrapy.downloadermiddlewares.redirect.MetaRefreshMiddleware',
'scrapy.downloadermiddlewares.httpcompression.HttpCompressionMiddleware',
'scrapy.downloadermiddlewares.redirect.RedirectMiddleware',
'scrapy.downloadermiddlewares.cookies.CookiesMiddleware',
'scrapy.downloadermiddlewares.chunked.ChunkedTransferMiddleware',
'scrapy.downloadermiddlewares.stats.DownloaderStats']
2016-06-21 22:45:46 [scrapy] INFO: Enabled spider middlewares:
['scrapy.spidermiddlewares.httperror.HttpErrorMiddleware',
'scrapy.spidermiddlewares.offsite.OffsiteMiddleware',
'scrapy.spidermiddlewares.referer.RefererMiddleware',
'scrapy.spidermiddlewares.urllength.UrlLengthMiddleware',
'scrapy.spidermiddlewares.depth.DepthMiddleware']
2016-06-21 22:45:46 [scrapy] INFO: Enabled item pipelines:
[]
2016-06-21 22:45:46 [scrapy] INFO: Spider opened
2016-06-21 22:45:46 [scrapy] INFO: Crawled 0 pages (at 0 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:48 [scrapy] INFO: Crawled 27 pages (at 1620 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:49 [scrapy] INFO: Crawled 59 pages (at 1920 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:50 [scrapy] INFO: Crawled 85 pages (at 1560 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:51 [scrapy] INFO: Crawled 123 pages (at 2280 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:52 [scrapy] INFO: Crawled 149 pages (at 1560 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:53 [scrapy] INFO: Crawled 181 pages (at 1920 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:54 [scrapy] INFO: Crawled 211 pages (at 1800 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:55 [scrapy] INFO: Crawled 237 pages (at 1560 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:56 [scrapy] INFO: Crawled 269 pages (at 1920 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:57 [scrapy] INFO: Closing spider (closespider_timeout)
2016-06-21 22:45:57 [scrapy] INFO: Crawled 307 pages (at 2280 pages/min), scraped 0 items (at 0 items/min)
2016-06-21 22:45:57 [scrapy] INFO: Dumping Scrapy stats:
{'downloader/request_bytes': 97844,
'downloader/request_count': 317,
'downloader/request_method_count/GET': 317,
'downloader/response_bytes': 469955,
'downloader/response_count': 317,
'downloader/response_status_count/200': 317,
'dupefilter/filtered': 204,
'finish_reason': 'closespider_timeout',
'finish_time': datetime.datetime(2016, 6, 21, 19, 45, 57, 835000),
'log_count/INFO': 17,
'request_depth_max': 14,
'response_received_count': 317,
'scheduler/dequeued': 317,
'scheduler/dequeued/memory': 317,
'scheduler/enqueued': 6136,
'scheduler/enqueued/memory': 6136,
'start_time': datetime.datetime(2016, 6, 21, 19, 45, 46, 986000)}
2016-06-21 22:45:57 [scrapy] INFO: Spider closed (closespider_timeout)
Into the next tutorial I will try to use scrapy.
If you have some ideas about how to do the next step just send me one comment.
Posted by
Cătălin George Feștilă
Labels:
2.7,
module,
pip,
pip2.7,
python,
scrapy,
windows,
winodws 10
Thursday, May 19, 2016
News: The new python version 3.6.0.a1
I used the Windows x86-64 executable installer to install this version of python.
I set some settings and I start the install aplication.
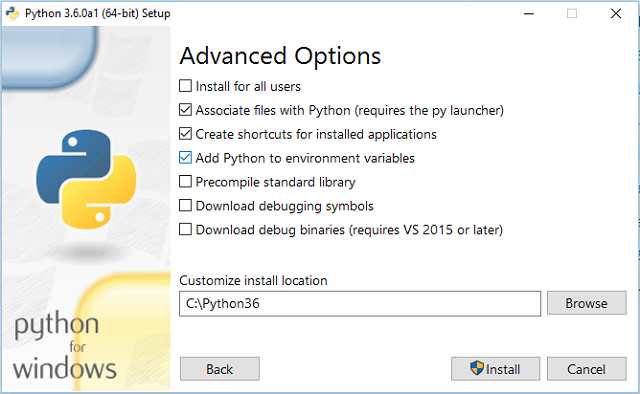
I read all new changes and PEP 0498.
I take a look to see all python modules:
I don't think the add Python start well, maybe need restart:
You can read more and also download the new python released version 360a1 from here.
Very good work from development team, they make a great job.
I set some settings and I start the install aplication.
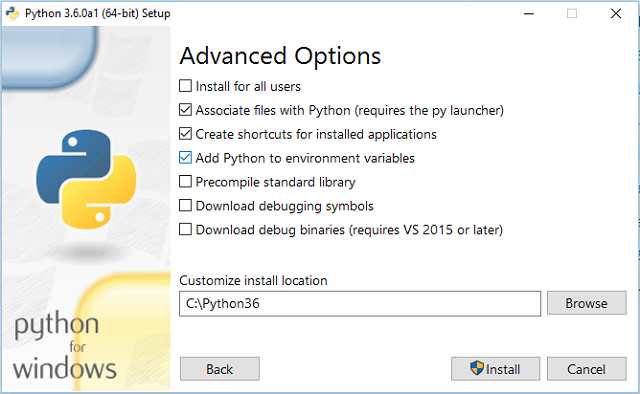
I read all new changes and PEP 0498.
I take a look to see all python modules:
Please wait a moment while I gather a list of all available modules...
__future__ aifc http setuptools
_ast antigravity idlelib shelve
_bisect argparse imaplib shlex
_bootlocale array imghdr shutil
_bz2 ast imp signal
_codecs asynchat importlib site
_codecs_cn asyncio inspect smtpd
_codecs_hk asyncore io smtplib
_codecs_iso2022 atexit ipaddress sndhdr
_codecs_jp audioop itertools socket
_codecs_kr base64 json socketserver
_codecs_tw bdb keyword sqlite3
_collections binascii lib2to3 sre_compile
_collections_abc binhex linecache sre_constants
_compat_pickle bisect locale sre_parse
_compression builtins logging ssl
_csv bz2 lzma stat
_ctypes cProfile macpath statistics
_ctypes_test calendar macurl2path string
_datetime cgi mailbox stringprep
_decimal cgitb mailcap struct
_dummy_thread chunk marshal subprocess
_elementtree cmath math sunau
_functools cmd mimetypes symbol
_hashlib code mmap symtable
_heapq codecs modulefinder sys
_imp codeop msilib sysconfig
_io collections msvcrt tabnanny
_json colorsys multiprocessing tarfile
_locale compileall netrc telnetlib
_lsprof concurrent nntplib tempfile
_lzma configparser nt test
_markupbase contextlib ntpath textwrap
_md5 copy nturl2path this
_msi copyreg numbers threading
_multibytecodec crypt opcode time
_multiprocessing csv operator timeit
_opcode ctypes optparse tkinter
_operator curses os token
_osx_support datetime parser tokenize
_overlapped dbm pathlib trace
_pickle decimal pdb traceback
_pydecimal difflib pickle tracemalloc
_pyio dis pickletools tty
_random distutils pip turtle
_sha1 doctest pipes turtledemo
_sha256 dummy_threading pkg_resources types
_sha512 easy_install pkgutil typing
_signal email platform unicodedata
_sitebuiltins encodings plistlib unittest
_socket ensurepip poplib urllib
_sqlite3 enum posixpath uu
_sre errno pprint uuid
_ssl faulthandler profile venv
_stat filecmp pstats warnings
_string fileinput pty wave
_strptime fnmatch py_compile weakref
_struct formatter pyclbr webbrowser
_symtable fractions pydoc winreg
_testbuffer ftplib pydoc_data winsound
_testcapi functools pyexpat wsgiref
_testimportmultiple gc queue xdrlib
_testmultiphase genericpath quopri xml
_thread getopt random xmlrpc
_threading_local getpass re xxsubtype
_tkinter gettext reprlib zipapp
_tracemalloc glob rlcompleter zipfile
_warnings gzip runpy zipimport
_weakref hashlib sched zlib
_weakrefset heapq secrets
_winapi hmac select
abc html selectors
The new formatted string literals are a new kind of string literal, prefixed with 'f' this allow you to add contain replacement fields surrounded by curly braces.I don't think the add Python start well, maybe need restart:
>>> import crypt
Traceback (most recent call last):
File "", line 1, in
File "C:\Python36\lib\crypt.py", line 3, in
import _crypt
ImportError: No module named '_crypt'
Some of changes can be see at whatsnew.You can read more and also download the new python released version 360a1 from here.
Very good work from development team, they make a great job.
Wednesday, April 20, 2016
News: New PyPy 5.1 released.
New features and bug fixes come with new PyPy 5.1 version.
The PyPy is a very compliant Python interpreter and supports x86 machines on most common operating systems.
Now we have full support for the IBM s390x and good optimizations.
Take a look here.
The PyPy is a very compliant Python interpreter and supports x86 machines on most common operating systems.
Now we have full support for the IBM s390x and good optimizations.
Take a look here.
Saturday, March 19, 2016
Free ebook from O'Reilly - Functional Programming in Python.
You can download your free ebook from O'Reilly.
The Functional Programming in Python by David Mertz - publisher: O'Reilly - released: June 2015.
David Mertz is a director of the Python Software Foundation, and chair of its Trademarks and Outreach & Education Committees. He wrote the columns Charming Python and XML Matters for IBM developerWorks and the Addison-Wesley book Text Processing in Python. David has spoken at multiple OSCON and PyCon events.
This is the download link.
The Functional Programming in Python by David Mertz - publisher: O'Reilly - released: June 2015.
David Mertz is a director of the Python Software Foundation, and chair of its Trademarks and Outreach & Education Committees. He wrote the columns Charming Python and XML Matters for IBM developerWorks and the Addison-Wesley book Text Processing in Python. David has spoken at multiple OSCON and PyCon events.
This is the download link.
Thursday, February 4, 2016
Testing PyQt4 under Python 3.5.1.
Today I working well with python 3.5.1.
Most of my source code was wrote with python 2.7 and the next step was to using pip3.5 to update and upgrade some python modules.
I try to install PyQt4 with pip3.5 - not working.
So I used the old way: whl file from here.
That was good, most of the scripts was running.
The main problem was OpenGL and that will make errors when you try to use QtOpenGL.
The result of this seam to me the python 3.5.1 it's now without OpenGL features.
Most of my source code was wrote with python 2.7 and the next step was to using pip3.5 to update and upgrade some python modules.
I try to install PyQt4 with pip3.5 - not working.
So I used the old way: whl file from here.
That was good, most of the scripts was running.
The main problem was OpenGL and that will make errors when you try to use QtOpenGL.
The result of this seam to me the python 3.5.1 it's now without OpenGL features.
The most short source code into Python 3.5.1 .
Just type this :
That will open your browser with one comics from xkcd website.
The antigravity module was added to Python 3.5.1. I'm not sure but seam working also with python 2.7 version.
import antigravity
That will open your browser with one comics from xkcd website.
The antigravity module was added to Python 3.5.1. I'm not sure but seam working also with python 2.7 version.
Saturday, January 23, 2016
wmi python module - part 002.
According to MSDN Microsoft the Win32_Process WMI class represents a process on an operating system.
We can see all of the inherited properties of processes:
We can see all of the inherited properties of processes:
class Win32_Process : CIM_Process
{
string Caption;
string CommandLine;
string CreationClassName;
datetime CreationDate;
string CSCreationClassName;
string CSName;
string Description;
string ExecutablePath;
uint16 ExecutionState;
string Handle;
uint32 HandleCount;
datetime InstallDate;
uint64 KernelModeTime;
uint32 MaximumWorkingSetSize;
uint32 MinimumWorkingSetSize;
string Name;
string OSCreationClassName;
string OSName;
uint64 OtherOperationCount;
uint64 OtherTransferCount;
uint32 PageFaults;
uint32 PageFileUsage;
uint32 ParentProcessId;
uint32 PeakPageFileUsage;
uint64 PeakVirtualSize;
uint32 PeakWorkingSetSize;
uint32 Priority = NULL;
uint64 PrivatePageCount;
uint32 ProcessId;
uint32 QuotaNonPagedPoolUsage;
uint32 QuotaPagedPoolUsage;
uint32 QuotaPeakNonPagedPoolUsage;
uint32 QuotaPeakPagedPoolUsage;
uint64 ReadOperationCount;
uint64 ReadTransferCount;
uint32 SessionId;
string Status;
datetime TerminationDate;
uint32 ThreadCount;
uint64 UserModeTime;
uint64 VirtualSize;
string WindowsVersion;
uint64 WorkingSetSize;
uint64 WriteOperationCount;
uint64 WriteTransferCount;
};
Let's make one simple example with wmi python module.import wmi
c = wmi.WMI()
for process in c.Win32_Process ():
name = process.Properties_("Name").Value
pid = process.Properties_('ProcessID').Value
parent = process.Properties_('ParentProcessId')
termination = process.Properties_('TerminationDate')
print (name,' = pid -',pid,'+', parent,'|termination_date-',termination)
And the output of this script it's :firefox.exe = pid - 13788 + 2564 |termination_date- None
explorer.exe = pid - 1048 + 772 |termination_date- None
sublime_text.exe = pid - 11404 + 2564 |termination_date- None
plugin_host.exe = pid - 7432 + 11404 |termination_date- None
cmd.exe = pid - 9568 + 2564 |termination_date- None
conhost.exe = pid - 14124 + 9568 |termination_date- None
conhost.exe = pid - 9700 + 11208 |termination_date- None
Taskmgr.exe = pid - 9424 + 13404 |termination_date- None
WmiPrvSE.exe = pid - 9764 + 772 |termination_date- None
SpfService64.exe = pid - 11908 + 684 |termination_date- None
python.exe = pid - 1308 + 9568 |termination_date- None
Posted by
Cătălin George Feștilă
Labels:
2016,
module,
python,
python 3,
python modules,
Spyder,
tutorial,
tutorials,
wmi
Friday, January 22, 2016
wmi python module - part 001.
Named WMI from Windows Management Instrumentation, this python module allow you to use Microsoft’s implementation of Web-Based Enterprise Management ( WBEM ).
Is a set of extensions to the Windows Driver Model AND that provides an operating system interface.
allows you to scripting languages like VBScript to manage Microsoft Windows personal computers and servers, both locally and remotely.
You cand read about this python module here.
Let try first example :
Now let's see another example can used by you with wmi python module.
This example let you see your processes.
I used the python version 3.3.5 and Spyder ( Scientific PYthon Development EnviRonment ) to test the script.
You can change .watch_for method args with: creation, deletion, modification or operation.
Is a set of extensions to the Windows Driver Model AND that provides an operating system interface.
allows you to scripting languages like VBScript to manage Microsoft Windows personal computers and servers, both locally and remotely.
You cand read about this python module here.
C:\Python34\Scripts>pip install wmi
...
Installing collected packages: wmi
Running setup.py install for wmi
warning: install_data: setup script did not provide a directory for 'readme.
txt' -- installing right in 'C:\Python34'
...
Successfully installed wmi
Cleaning up...
Let try first example :
C:\Python34>python
Python 3.4.1 (v3.4.1:c0e311e010fc, May 18 2014, 10:45:13) [MSC v.1600 64 bit (AM
D64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import wmi
>>> remote_process = wmi.WMI (computer="home").new ("Win32_Process")
>>> for i in wmi.WMI ().Win32_OperatingSystem ():
... print (i.Caption)
...
Microsoft Windows 10 Home
Now let's see another example can used by you with wmi python module.
This example let you see your processes.
import wmi
import datetime
c = wmi.WMI()
process_watcher = c.Win32_Process.watch_for("modification")
while True:
new_process = process_watcher()
print (new_process.Caption)
I used the python version 3.3.5 and Spyder ( Scientific PYthon Development EnviRonment ) to test the script.
You can change .watch_for method args with: creation, deletion, modification or operation.
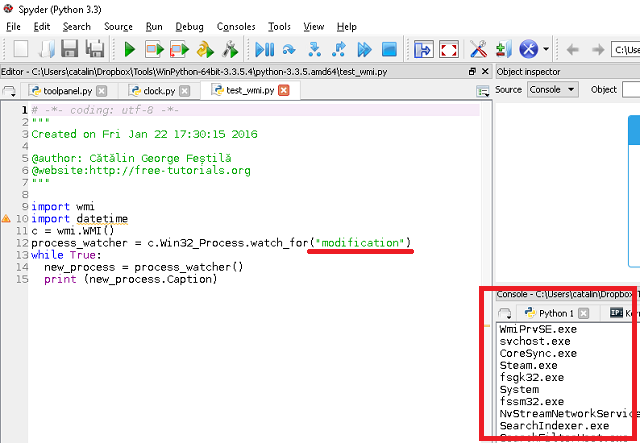
Posted by
Cătălin George Feștilă
Labels:
2016,
module,
python,
python 3,
python modules,
Spyder,
tutorial,
tutorials,
wmi
Saturday, November 28, 2015
The svgwrite python module - part 001.
The svgwrite python module is a tool by Manfred Moitzi.
To install this python module use Python 3.4 version.
Then you need to install svgwrite with pip3.4:
See this simple example:
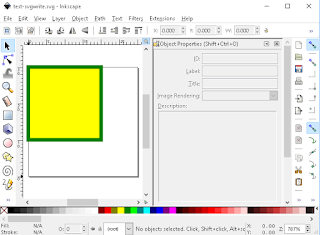
If you use the dir() then you can see more about this python module:
To install this python module use Python 3.4 version.
Then you need to install svgwrite with pip3.4:
C:\Python34\Scripts>pip3.4.exe install svgwrite
Collecting svgwrite
Downloading svgwrite-1.1.6.tar.gz (109kB)
100% |################################| 110kB 354kB/s
Also this svgwrite is the only module that needs to be imported and then you can deal with this module.See this simple example:
import svgwrite
from svgwrite import *
#this will work with svgwrite
svg_doc = svgwrite.Drawing(filename = "test-svgwrite.svg",\
size = ("30px", "30px"))\
svg_doc.add(svg_doc.rect(insert = (0, 0),\
size = ("20px", "20px"),\
stroke_width = "1",\
stroke = "green",\
fill = "rgb(255,255,0)"))\
print(svg_doc.tostring())
svg_doc.save()
The result of this python script is this SVG file.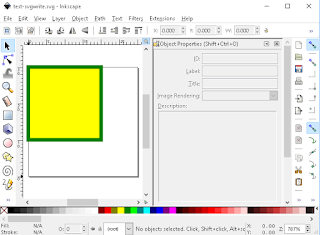
If you use the dir() then you can see more about this python module:
>>> dir(svgwrite)
['AUTHOR_EMAIL', 'AUTHOR_NAME', 'CYEAR', 'Drawing', 'Hz', 'Unit', 'VERSION', '__
builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__p
ackage__', '__path__', '__spec__', 'animate', 'base', 'cm', 'container', 'data',
'deg', 'drawing', 'elementfactory', 'em', 'etree', 'ex', 'filters', 'grad', 'gr
adients', 'image', 'inch', 'kHz', 'masking', 'mixins', 'mm', 'params', 'path', '
pattern', 'pc', 'percent', 'pt', 'px', 'rad', 'rgb', 'shapes', 'text', 'utils',
'validator2', 'version']
For example you can add some text:svg_doc.add(svg_document.text("This add new svg text",\
insert = (10, 10)))
Posted by
Cătălin George Feștilă
Labels:
2015,
python 3,
python modules,
svgwrite,
tutorial,
tutorials
Sunday, November 22, 2015
The new Python 2.7.11rc1.
The next release in the 2.7.x series is now available with the version 2.7.11.rc.1 .
This can be download it from here.
Come with source release, installer for Windows, Mac OS X 10.6 and later.
I don't use linux instalation, just on Windows 10 - 64 MSI installer.
The changes of this version can be see here.
First I saw this python version will change my old python 2.7 version.
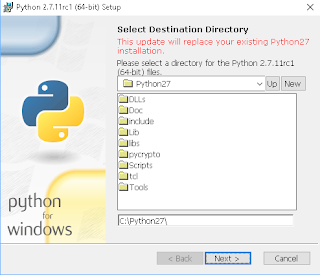
Also I check to compile all python scripts and module.
With installed Python 2.7 I check if is all ok.
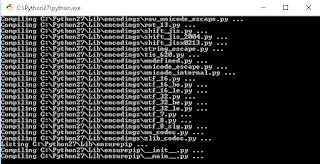
I think the python has some problems with keep and clean correct modules under pip.
Also I guest can be done with few rows of python scripts but also this can be done by default by python installation.
This can be download it from here.
Come with source release, installer for Windows, Mac OS X 10.6 and later.
I don't use linux instalation, just on Windows 10 - 64 MSI installer.
The changes of this version can be see here.
First I saw this python version will change my old python 2.7 version.
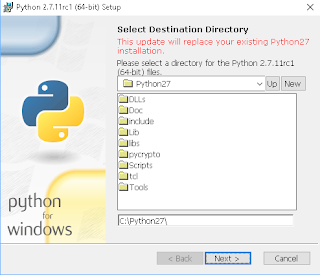
Also I check to compile all python scripts and module.
With installed Python 2.7 I check if is all ok.
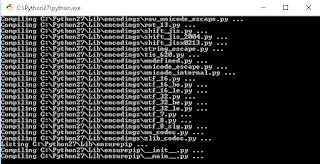
C:\Python27>python.exe
Python 2.7.11rc1 (v2.7.11rc1:82dd9545bd93, Nov 21 2015, 23:25:27) [MSC v.1500 64
bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>
Also I got a list of all available modules...No handlers could be found for logger "OpenGL.Tk"
ImportError: numpy.core.multiarray failed to import
BaseHTTPServer audioop imaplib scrap
Bastion base imghdr select
BeautifulSoup base64 imp sets
BeautifulSoupTests bdb importlib setuptools
CGIHTTPServer binascii imputil sgmllib
Canvas binhex inspect sha
ConfigParser bisect io shellescape
Cookie bs4 itertools shelve
Dialog bsddb joystick shelve_test
DocXMLRPCServer bufferproxy json shlex
FileDialog bz2 key shutil
FixTk cPickle keyword signal
HTMLParser cProfile lib2to3 simplejson
MimeWriter cStringIO linecache site
Naked cached_property locale six
OpenGL calendar locals smtpd
PIL camera logging smtplib
PySide cdrom mac_scrap sndarray
Queue cgi macosx sndhdr
ScrolledText cgitb macpath socket
SimpleDialog chunk macurl2path sprite
SimpleHTTPServer cmath mailbox sqlite3
SimpleXMLRPCServer cmd mailcap sre
SocketServer code markupbase sre_compile
StringIO codecs marshal sre_constants
Tix codeop mask sre_parse
Tkconstants collections math ssl
Tkdnd color md5 stat
Tkinter colorama mhlib statvfs
UserDict colordict midi string
UserList colorsys mimetools stringold
UserString commands mimetypes stringprep
_LWPCookieJar compat mimify strop
_MozillaCookieJar compileall mixer struct
__builtin__ compiler mixer_music subprocess
__future__ constants mmap sunau
_abcoll contextlib modulefinder sunaudio
_arraysurfarray cookielib mouse surface
_ast copy movie surfarray
_bisect copy_reg msilib surflock
_bsddb crypto msvcrt symbol
_camera csv multifile symtable
_camera_opencv_highgui ctypes multiprocessing sys
_camera_vidcapture curses mutex sysconfig
_codecs cursors netrc sysfont
_codecs_cn cv new tabnanny
_codecs_hk cv2 newbuffer tarfile
_codecs_iso2022 datetime nmovie telnetlib
_codecs_jp dateutil nntplib tempfile
_codecs_kr dbhash nt test
_codecs_tw decimal ntpath test27
_collections decorator nturl2path test_crypto_python
_csv difflib numbers tests
_ctypes dircache numpy textwrap
_ctypes_test dis oauth2client this
_dummybackend display opcode thread
_elementtree distutils operator threading
_freetype docs optparse threads
_functools doctest os time
_hashlib draw os2emxpath timeit
_heapq dumbdbm overlay tkColorChooser
_hotshot dummy_thread parser tkCommonDialog
_io dummy_threading pdb tkFileDialog
_json easy_install pickle tkFont
_locale ecdsa pickletools tkMessageBox
_lsprof email pip tkSimpleDialog
_markerlib encodings pipes toaiff
_md5 ensurepip pixelarray token
_msi errno pixelcopy tokenize
_multibytecodec event pkg_resources trace
_multiprocessing examples pkgdata traceback
_numericsndarray exceptions pkgutil transform
_numericsurfarray extraction platform ttk
_numpysndarray fastevent plistlib tty
_numpysurfarray filecmp popen2 turtle
_osx_support fileinput poplib types
_pyio fnmatch posixfile unicodedata
_pytest font posixpath unittest
_random formatter pprint uritemplate
_sha fpformat profile url-scrap
_sha256 fractions pstats urllib
_sha512 freetype pty urllib2
_socket ftfont py urllib3
_sqlite3 ftplib py_compile urlparse
_sre functools pyasn1 urwid
_ssl future_builtins pyasn1_modules user
_strptime gc pyclbr uu
_struct genericpath pycuda uuid
_subprocess get-pip pydoc version
_symtable getopt pydoc_data vidcap
_testcapi getpass pyexpat virtualenv
_threading_local gettext pygame virtualenv_support
_tkinter gfxdraw pypm warnings
_vlcbackend glob pysideuic wave
_warnings googleapiclient pytest weakref
_weakref gp2x pytools webbrowser
_weakrefset gzip pytz wheel
_winreg hashlib quopri whichdb
abc heapq random winsound
aifc hmac re wsgiref
antigravity hotshot rect xdrlib
anydbm html5lib repr xlsxwriter
apiclient htmlentitydefs requests xml
appdirs htmllib rexec xmllib
argparse httplib rfc822 xmlrpclib
array httplib2 rlcompleter xxsubtype
ast idlelib robotparser yaml
asynchat ihooks rsa yt
asyncore image runpy zipfile
atexit imageext rwobject zipimport
audiodev imageop sched zlib
Enter any module name to get more help. Or, type "modules spam" to search
for modules whose descriptions contain the word "spam".
I update some module with pip2.7 C:\Python27\Scripts>C:\Python27\python.exe
Python 2.7.11rc1 (v2.7.11rc1:82dd9545bd93, Nov 21 2015, 23:25:27) [MSC v.1500 64
bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import pip
>>> from subprocess import call
>>>
>>> for dist in pip.get_installed_distributions():
... call("pip2.7.exe install --upgrade " + dist.project_name, shell=True)
...
Requirement already up-to-date: appdirs in c:\python27\lib\site-packages
0
Requirement already up-to-date: BeautifulSoup in c:\python27\lib\site-packages
0
Collecting beautifulsoup4
Downloading beautifulsoup4-4.4.1-py2-none-any.whl (81kB)
100% |################################| 81kB 327kB/s
Installing collected packages: beautifulsoup4
Found existing installation: beautifulsoup4 4.3.2
Uninstalling beautifulsoup4-4.3.2:
Successfully uninstalled beautifulsoup4-4.3.2
Successfully installed beautifulsoup4-4.4.1
0
Some python modules has problems with update...
Failed building wheel for pycuda
Note:I think the python has some problems with keep and clean correct modules under pip.
Also I guest can be done with few rows of python scripts but also this can be done by default by python installation.
Subscribe to:
Posts (Atom)