In this article tutorial I will show you how to read from the sqlite file the content of the table: files.
In the last article tutorial, I create a interface with PyQt6 that search files by regular expresion and result is add to sqlite file named: file_paths.db.
I used same steps with a default python class and I used QSqlTableModel to show the content received.
The script will create a window with this QSqlTableModel, then reads the file and add the result.
Let's see the source code:
import sys
from PyQt6.QtWidgets import QApplication, QMainWindow, QTableView
from PyQt6.QtSql import QSqlDatabase, QSqlQuery, QSqlTableModel
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# Initialize the database
self.init_db()
# Set up the GUI
self.table_view = QTableView(self)
self.setCentralWidget(self.table_view)
# Set up the model and connect it to the database
self.model = QSqlTableModel(self)
self.model.setTable('files')
self.model.select()
self.table_view.setModel(self.model)
def init_db(self):
# Connect to the database
db = QSqlDatabase.addDatabase('QSQLITE')
db.setDatabaseName('file_paths.db')
if not db.open():
print('Could not open database')
sys.exit(1)
def create_table(self):
# Create the 'files' table if it doesn't exist
query = QSqlQuery()
query.exec('CREATE TABLE IF NOT EXISTS files (id INTEGER PRIMARY KEY, path TEXT)')
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec())
I add this source code into a python script named view.py and I run it.
This is the result of the running script:
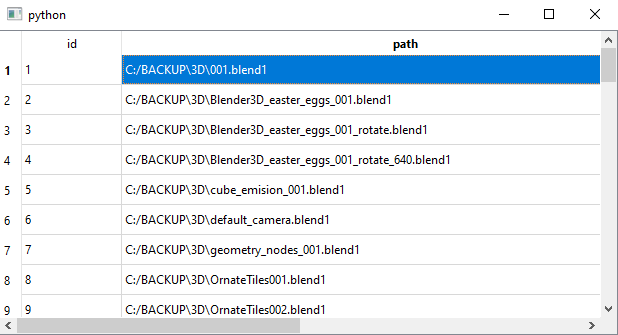