In this tutorial, I will show you how easy it is to work with PyQt6 and QTableWidget to display any CSV file.
The source code lines are already commented to understand how this source code works.
This is the content of the csv file named my.csv:
id,firstname,lastname,email,email2,profession
100,Maud,Callista,Maud.Callista@yopmail.com,Maud.Callista@gmail.com,police officer
101,Justinn,Rona,Justinn.Rona@yopmail.com,Justinn.Rona@gmail.com,worker
102,Gabriellia,Robertson,Gabriellia.Robertson@yopmail.com,Gabriellia.Robertson@gmail.com,police officer
103,Gwenneth,Payson,Gwenneth.Payson@yopmail.com,Gwenneth.Payson@gmail.com,firefighter
104,Lynea,Robertson,Lynea.Robertson@yopmail.com,Lynea.Robertson@gmail.com,developer
This is the source I used:
import sys
import csv
from PyQt6.QtWidgets import QApplication, QMainWindow, QTableWidget, QTableWidgetItem
from PyQt6.QtCore import Qt
# the main window class
class MainWindow(QMainWindow):
# the init definition of the class
def __init__(self):
super().__init__()
# the window title
self.setWindowTitle('Table Viewer for any CSV file.')
# this create the table widget
self.table = QTableWidget(self)
# the table dimensions is set default
self.table.setColumnCount(0)
self.table.setRowCount(0)
# this read the CSV file named my.csv
with open('my.csv', 'r') as file:
# use the reader for file
reader = csv.reader(file)
# this get the column labels from the first row
headers = next(reader)
# this set the number of columns based on the number of headers
self.table.setColumnCount(len(headers))
# this set the horizontal header labels
self.table.setHorizontalHeaderLabels(headers)
# for each row iterate the rows in the CSV file
for row in reader:
# add a row to the table
row_index = self.table.rowCount()
self.table.insertRow(row_index)
# add data to cells
for col_index, cell in enumerate(row):
self.table.setItem(row_index, col_index, QTableWidgetItem(cell))
# setting for the table as the central widget
self.setCentralWidget(self.table)
# this run the application
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec())
Here is what the result of running the source code:
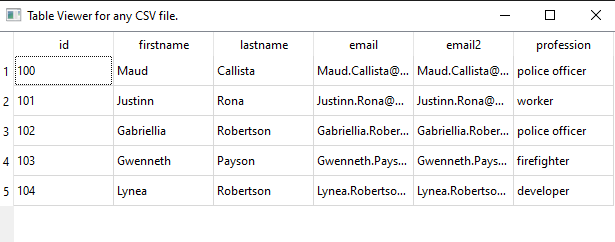