First I create tree scripts named:
- PyQt5_connection.py - create a memory database and add value into table;
- PyQt5_view.py - create a model for the table;
- PyQt5_show.py - show the MDI application with the model table database;
Let's see this python scripts:
First is PyQt5_connection.py:
from PyQt5 import QtWidgets, QtSql
from PyQt5.QtSql import *
def createConnection():
db = QtSql.QSqlDatabase.addDatabase("QSQLITE")
db.setDatabaseName(":memory:")
if not db.open():
QtWidgets.QMessageBox.critical(None, "Cannot open memory database",
"Unable to establish a database connection.\n\n"
"Click Cancel to exit.", QtWidgets.QMessageBox.Cancel)
return False
query = QtSql.QSqlQuery()
#print (os.listdir("."))
query.exec("DROP TABLE IF EXISTS Websites")
query.exec("CREATE TABLE Websites (ID INTEGER PRIMARY KEY NOT NULL, " + "website VARCHAR(20))")
query.exec("INSERT INTO Websites (website) VALUES('python-catalin.blogspot.com')")
query.exec("INSERT INTO Websites (website) VALUES('catalin-festila.blogspot.com')")
query.exec("INSERT INTO Websites (website) VALUES('free-tutorials.org')")
query.exec("INSERT INTO Websites (website) VALUES('graphic-3d.blogspot.com')")
query.exec("INSERT INTO Websites (website) VALUES('pygame-catalin.blogspot.com')")
return True
The next is the PyQt5_view.py python script with the model of the table from database:from PyQt5 import QtWidgets, QtSql
from PyQt5 import QtWidgets, QtSql
class WebsitesWidget(QtWidgets.QWidget):
def __init__(self, parent=None):
super(WebsitesWidget, self).__init__(parent)
# this layout_box can be used if you need more widgets
# I used just one named WebsitesWidget
layout_box = QtWidgets.QVBoxLayout(self)
#
my_view = QtWidgets.QTableView()
# put viwe in layout_box area
layout_box.addWidget(my_view)
# create a table model
my_model = QtSql.QSqlTableModel(self)
my_model.setTable("Websites")
my_model.select()
#show the view with model
my_view.setModel(my_model)
my_view.setItemDelegate(QtSql.QSqlRelationalDelegate(my_view))
The last python script named PyQt5_show.py will create the MDI application and will show databese table:from PyQt5 import QtWidgets, QtSql
from PyQt5_connection import createConnection
# this will import any classes from PyQt5_view script
from PyQt5_view import WebsitesWidget
class MainWindow(QtWidgets.QMainWindow):
def __init__(self, parent=None):
super(MainWindow, self).__init__(parent)
self.MDI = QtWidgets.QMdiArea()
self.setCentralWidget(self.MDI)
SubWindow1 = QtWidgets.QMdiSubWindow()
SubWindow1.setWidget(WebsitesWidget())
self.MDI.addSubWindow(SubWindow1)
SubWindow1.show()
# you can add more widgest
#SubWindow2 = QtWidgets.QMdiSubWindow()
if __name__ == '__main__':
import sys
app = QtWidgets.QApplication(sys.argv)
if not createConnection():
print("not connect")
sys.exit(-1)
w = MainWindow()
w.show()
sys.exit(app.exec_())
This is the result of the running PyQt5_show.py python script: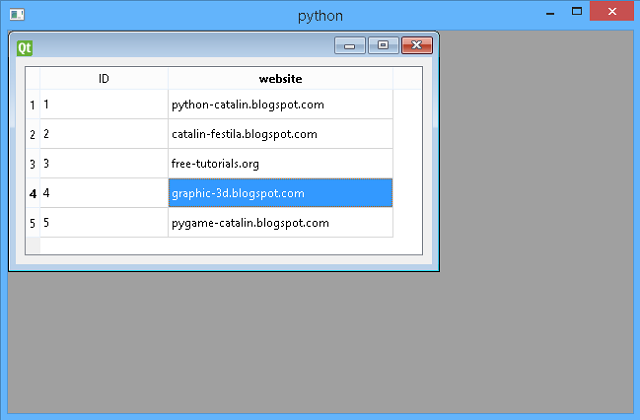