Let's install it under Windows 10.
C:\Python27\Scripts>pip install pythonnet
Collecting pythonnet
Downloading pythonnet-2.3.0-cp27-cp27m-win32.whl (58kB)
100% |################################| 61kB 740kB/s
Installing collected packages: pythonnet
Successfully installed pythonnet-2.3.0
Now I will show you how to use form and buttons.First, you need to run the python code into python script files.
The first example is simple:
import clr
clr.AddReference("System.Windows.Forms")
from System.Windows.Forms import Application, Form
class IForm(Form):
def __init__(self):
self.Text = 'Simple'
self.Width = 640
self.Height = 480
self.CenterToScreen()
Application.Run(IForm())
The next example comes with one button and tooltips for form and button:import clr
clr.AddReference("System.Windows.Forms")
clr.AddReference("System.Drawing")
from System.Windows.Forms import Application, Form
from System.Windows.Forms import Button, ToolTip
from System.Drawing import Point, Size
class IForm(Form):
def __init__(self):
self.Text = 'Tooltips'
self.CenterToScreen()
self.Size = Size(640, 480)
tooltip = ToolTip()
tooltip.SetToolTip(self, "This is a Form")
button = Button()
button.Parent = self
button.Text = "Button"
button.Location = Point(50, 70)
tooltip.SetToolTip(button, "This is a Button")
Application.Run(IForm())
This is the result of this python script.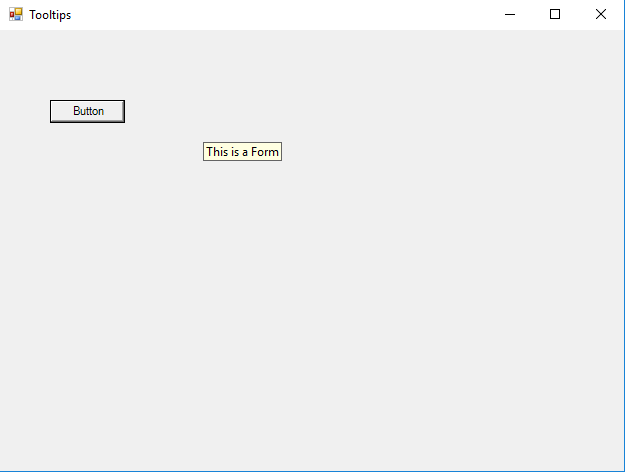
Another example is how to see the interfaces that are part of a .NET assembly:
>>> import System.Collections
>>> interfaces = [entry for entry in dir(System.Collections)
... if entry.startswith('I')]
>>> for entry in interfaces:
... print entry
...
ICollection
IComparer
IDictionary
IDictionaryEnumerator
IEnumerable
IEnumerator
IEqualityComparer
IHashCodeProvider
IList
IStructuralComparable
IStructuralEquatable