I used the python version 3.6.4 to test the last Django framework version.
Add your python to the path environment variable under Windows O.S.
Create your working folder:
C:\Python364>mkdir mywebsite
Go to the folder to install all you need:
C:\Python364>cd mywebsite
Use a virtual environment using the virtualenv command:
C:\Python364\mywebsite>python -m venv myvenv
C:\Python364\mywebsite>myvenv\Scripts\activate
(myvenv) C:\Python364\mywebsite>python -m pip install --upgrade pip
(myvenv) C:\Python364\mywebsite>pip3.6 install django
Collecting django
...
If you try to run again this command you will see the version of Django:
(myvenv) C:\Python364\mywebsite>pip3.6 install django
Requirement already satisfied: django in c:\python364\mywebsite\myvenv\lib\
site-packages (2.1.1)
Requirement already satisfied: pytz in c:\python364\mywebsite\myvenv\lib\
site-packages (from django) (2018.5)
You need to run the django-admin command:
(myvenv) C:\Python364\mywebsite>cd myvenv
(myvenv) C:\Python364\mywebsite\myvenv>cd Scripts
(myvenv) C:\Python364\mywebsite\myvenv\Scripts>django-admin.exe startproject mysite
(myvenv) C:\Python364\mywebsite\myvenv\Scripts>dir my*
(myvenv) C:\Python364\mywebsite\myvenv\Scripts>cd mysite
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite&
Make a change to settings file:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>cd mysite
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\mysite>notepad settings.py
Change UTC timezone:
TIME_ZONE = 'Europe/Paris'
Change host:
ALLOWED_HOSTS = ['192.168.0.185','mysite.com']
The next step is to use these commands:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\mysite>cd ..
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py migrate
Operations to perform:
Apply all migrations: admin, auth, contenttypes, sessions
Running migrations:
Applying contenttypes.0001_initial... OK
Applying auth.0001_initial... OK
Applying admin.0001_initial... OK
Applying admin.0002_logentry_remove_auto_add... OK
Applying admin.0003_logentry_add_action_flag_choices... OK
Applying contenttypes.0002_remove_content_type_name... OK
Applying auth.0002_alter_permission_name_max_length... OK
Applying auth.0003_alter_user_email_max_length... OK
Applying auth.0004_alter_user_username_opts... OK
Applying auth.0005_alter_user_last_login_null... OK
Applying auth.0006_require_contenttypes_0002... OK
Applying auth.0007_alter_validators_add_error_messages... OK
Applying auth.0008_alter_user_username_max_length... OK
Applying auth.0009_alter_user_last_name_max_length... OK
Applying sessions.0001_initial... OK
Let's try these steps with the browser:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py runserver
192.168.0.185:8080
Performing system checks...
System check identified no issues (0 silenced).
September 07, 2018 - 16:30:13
Django version 2.1.1, using settings 'mysite.settings'
Starting development server at http://192.168.0.185:8080/
Quit the server with CTRL-BREAK.
[07/Sep/2018 16:30:16] "GET / HTTP/1.1" 200 16348
[07/Sep/2018 16:30:21] "GET / HTTP/1.1" 200 16348
This is the result:
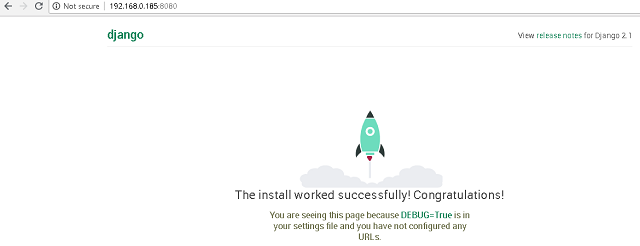
Let's start Django application named
myblog and add to settings.py :
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py startapp
myblog
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>dir
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>cd mysite
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\mysite>notepad settings.py
Search into settings.py this line and add 'myblog' and comma after, see:
# Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'myblog',
]
Let's change
models.py from
myblog folder:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\mysite>cd ..
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>cd myblog
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\myblog>notepad models.py
Add this source code:
from django.db import models
# Create your models here.
from django.utils import timezone
from django.contrib.auth.models import User
class Post(models.Model):
author = models.ForeignKey(User,on_delete=models.PROTECT)
title = models.CharField(max_length=200)
text = models.TextField()
create_date = models.DateTimeField(default=timezone.now)
published_date = models.DateTimeField(blank=True, null=True)
def publish(self):
self.publish_date = timezone.now()
self.save()
def __str__(self):
return self.title
Go and run this command manage.py for model Post with
makemigrations myblog and
migrate myblog :
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\myblog>cd ..
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py
makemigrations myblog
Migrations for 'myblog':
myblog\migrations\0001_initial.py
- Create model Post
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py migrate
myblog
Operations to perform:
Apply all migrations: myblog
Running migrations:
Applying myblog.0001_initial... OK
Add this source code to
admin.py from
myblog folder:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>cd myblog
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\myblog>notepad admin.py
Let's test again:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite\myblog>cd ..
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py runserver
192.168.0.185:8080
Performing system checks...
System check identified no issues (0 silenced).
September 07, 2018 - 17:19:00
Django version 2.1.1, using settings 'mysite.settings'
Starting development server at http://192.168.0.185:8080/
Quit the server with CTRL-BREAK.
Check the admin interface with add admin word to link, see: http://192.168.0.185:8080/admin
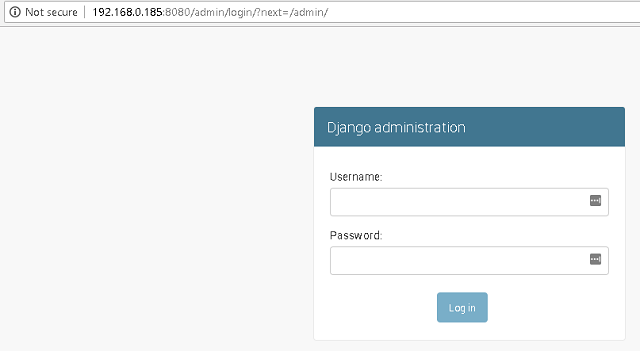
If you see some errors this will be fixed later.
Let's make a superuser with this command:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py
createsuperuser
Username (leave blank to use 'catafest'): catafest
Email address: catafest@yahoo.com
Password:
Password (again):
This password is too short. It must contain at least 8 characters.
Bypass password validation and create user anyway? [y/N]: y
Superuser created successfully.
Run again this command and log in with your user and password:
(myvenv) C:\Python364\mywebsite\myvenv\Scripts\mysite>python manage.py runserver
192.168.0.185:8080
This is the result of users and posts.
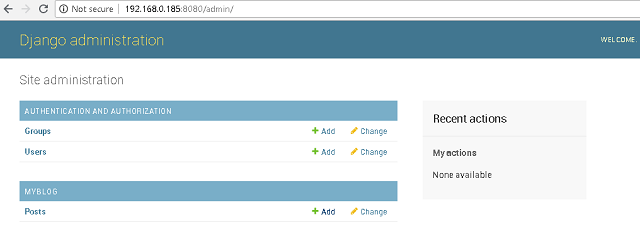
Click on the
Add button from
Posts to add your post.
The result is this:
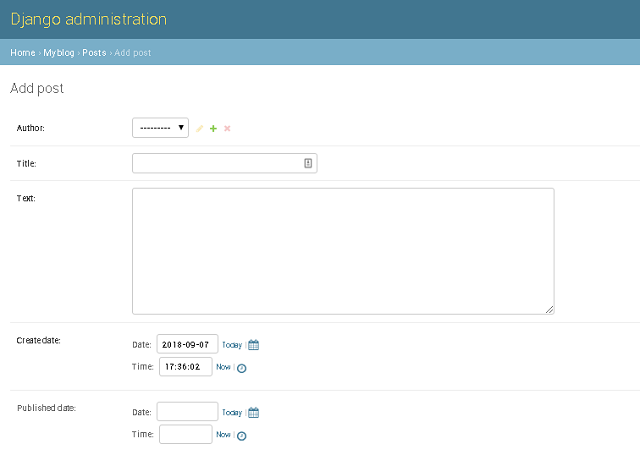
I don't make settings for URL and view.
This will be changed by users.